In this I need to write a file in android's internal storage so that i can acccess it. I have given Write to External Storage permissions but when I try to write the file it says Access is denied.
First of all, the permission <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
is for external storage, hence it doesn't work for the internal storage.
Based on your code:
Java.IO.File DataDirectoryPath = Android.OS.Environment.DataDirectory;
You used Data Directory
for your writing, this directory is owned by system and there is no permission for writing to it, there're several discussions about this issue on SO, for example: Data directory has no read/write permission in Android
.
So, as it suggested in the answer there, you can use getFilesDir()
to write in internal storage, in Xamarin, it is Android.Content.Context.FilesDir Property.
Update:
I wrote some code based on your code, it should be the same, for example:
var dirPath = this.FilesDir + "/MyFolder";
var exists = Directory.Exists(dirPath);
var filepath = dirPath + "/test.txt";
if (!exists)
{
Directory.CreateDirectory(dirPath);
if (!System.IO.File.Exists(filepath))
{
var newfile = new Java.IO.File(dirPath, "test.txt");
using (FileOutputStream outfile = new FileOutputStream(newfile))
{
string line = "The very first line!";
outfile.Write(System.Text.Encoding.ASCII.GetBytes(line));
outfile.Close();
}
}
}
I also checked this file by DDMS, it can be seen under the folder: data\data\yourAPP\files\MyFolder\test.txt
:
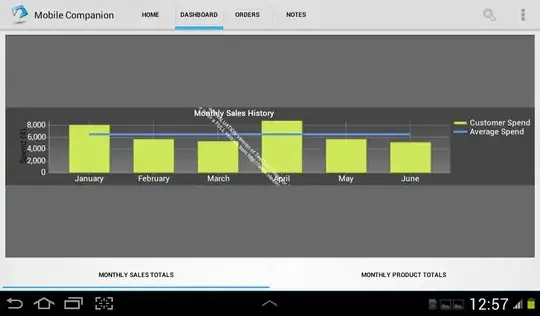
I just test Xamarin.Android project, didn't tried to use DependencyService
for Xamarin.Forms, if you're trying to do that, just replace the word this
in the code above to your android context.