First of all Few things to notice:
First get some idea about access modifiers:
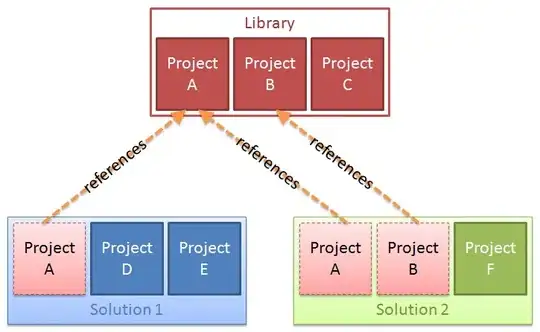
About private constructor:
prevent subclassing (extending). If you make only a private constructor(only have one constructor), no class can extend your class, because it can't call the super()
constructor. read more
And little bit about constructor:
- Constructors are never inherited - they are specific to the class in
which they are defined.
- If no constructor implemented, default constructor will added by the
compiler implicitly.
- In subclasses did not call the
super()
compiler automatically add those to all the constructors.
- When a new object that is a subclass is constructed, the constructor
for the superclass is always called first.
- Constructor invocation maybe implicit or explicit.
- explicit call to a constructor of superclass:
super()
- has to be the first statement in the subclass constructor.
Without any constructor in MG
:
In this line public class MG extends Car {
eclipse show error:
Implicit super constructor Car()
is not visible for default
constructor. Must define an explicit constructor.
Because of inheritance you need to pass values to parent class constructor.
And for this new MG(7);
:
The constructor MG(int) is undefined
Because you donot have any parameterized constructor in MG
class.
UPDATE:
You said in your question you only want to know about line number 7, 11, 12, 13 and 14. But I will answer for all the lines. Then others who looking for answer also can understand
If you uncomment line number 7:
When we create an object of a child class always the constructor of the parent get completed and the constructor of the child runs later.
When you run the program, First in the main method new MG(7);
object looking for matching constructor in the MG
, which is MG(int x) {}
. Inside this has super()
which is compiler added one. Then compiler look for default constructor in parent class, It cannot find since default constructor is private
.
To fix this: MG(int x) {super(x);}
If you uncomment line number 8:
When you run the program, First in the main method new MG(7);
object looking for matching constructor in the MG
, which is found.
MG(int x) {super()}
If you added super()
or not compiler will add this. There is no different between these two:
MG(int x) {}
MG(int x) {super()}
If you uncomment line number 9:
This line is fine(no errors). First compiler looking for constructor(MG(int x) { super(x); }
for object(new MG(7);
). Then inside the constructor have called super(x)
which is have one argument and it is match with the parent class constructor. As I mentioned before, parent class constructor execute first, Then child class constructor execute.
If you uncomment line number 10:
private MG(int x) { super(x); }
Access modifier is not affected because this class is a subclass and it is not a parent class for any class. And we can create object from calling private
constructor. read more about private constructor.
If you uncomment line number 11:
MG() { }
It is match with the new MG();
object. Compiler have added super()
but no matching constructor found in the parent class.
If you uncomment line number 12:
MG() { this(); }
First, lets look at what is this()
:
this
can be used inside the Method or constructor of Class.
this
works as a reference to the current Object whose Method or
constructor is being invoked.
- The this keyword can be used to refer to any member of the current
object from within an instance Method or a constructor.
this()
keyword can be used inside the constructor to call another
overloaded constructor in the same Class.
- This is also called the Explicit Constructor Invocation.
- And
this()
and super()
both cannot use in one constructor.
So this line is unnecessary because you don't have any other MG()
constructors. learn more about this
.
If you uncomment line number 13:
MG() { this(6); }
This is also unnecessary or cannot be called unless you have another MG()
constructor. If you do like this:
MG(){
this(6);
}
MG(int x){
super(x);
}
What happen here is when you run the program new MG();
object find the default constructor and in it call the parameterized constructor(MG(int x){}
). Then in the this constructor take the value and send it to the parent class.
If you uncomment line number 14:
MG() { super(7); }
new MG();
is matched with the costructor and in it you have passed value 7
to the parent class parameterized constructor.
Done.