It's better not to use Reset Transformations
command for your Cone
from Modify
main menu, 'cause pivot point goes back to its initial place. The analog for useful Freeze Transformations
command in Python is cmds.makeIdentity()
. Even if you've moved you pivot point by 1
unit (for example) along +Y
axis, do not forget to subtract that 1
from variable y
cause Maya somehow remembers pivot's position. Offset of the Cone's pivot point (for snapping pivot to a vertex) depends on the Cone's size itself. By default it's 1
.
Add this snippet to your code to move the duplicates in World Space
:
# cmds.makeIdentity( 'pCone1', apply=True )
pivSnap = 1
cmds.xform( 'pCone1', piv=[ 0, pivSnap, 0 ] )
cmds.move( x, y-pivSnap, z, a=True, ws=True, wd=True )
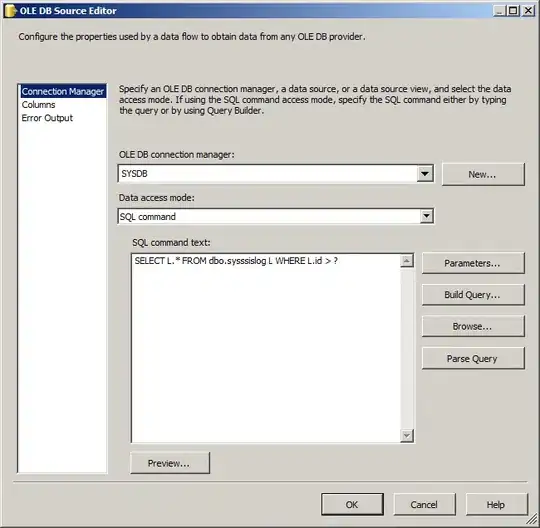
You can test this code (here I moved pivot up by 0.5
):
import maya.cmds as cmds
cmds.polyCube( sx=1, sy=15, sz=1, w=1, h=15, d=1 )
cmds.polyCone( r=.5, h=1, sx=10 )
cmds.move( 10, x=True )
pivSnap = .5
cmds.xform( 'pCone1', piv=[ 0, pivSnap, 0 ] )
cmds.rotate( 0, 0, '45deg' )
cmds.select( 'pCube1.e[33]','pCube1.e[37]','pCube1.e[41]','pCube1.e[45]','pCube1.e[49]','pCube1.e[53]','pCube1.e[57]','pCube1.e[61]' )
vertices = []
srcObj = "pCone1"
def snapToVertex( vertex, object ):
cmds.select( vertex )
x,y,z = cmds.pointPosition()
print( x,y,z )
cmds.select( object )
cmds.manipMoveContext( m=2 )
cmds.delete( 'pCube1', ch=True )
cmds.duplicate()
cmds.move( x, y-pivSnap, z, a=True, ws=True, wd=True )
def processTask():
cmds.select( cmds.polyListComponentConversion( tv=True ) )
vertices = cmds.ls( sl=True )
print( vertices )
for vrtx in vertices:
snapToVertex( vrtx, srcObj )
processTask()
