In short you can accomplish modal validation by utilizing the native gii generated activeforms using the following steps:
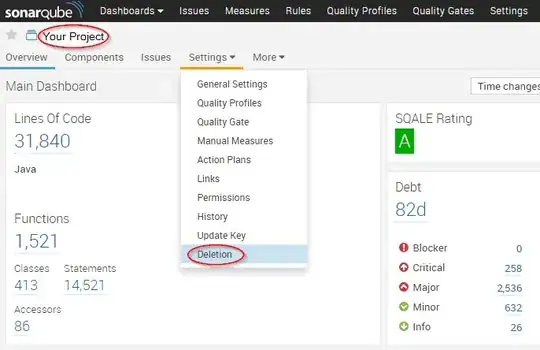
Create File at Location: app/web/js/ajax-modal-popup.js
$(function(){
$(document).on('click', '.showModalButton', function(){
if ($('#modal').data('bs.modal').isShown) {
$('#modal').find('#modalContent')
.load($(this).attr('value'));
document.getElementById('modalHeader').innerHTML = '<h4>' + $(this).attr('title') + '</h4>';
} else {
$('#modal').modal('show')
.find('#modalContent')
.load($(this).attr('value'));
document.getElementById('modalHeader').innerHTML = '<h4>' + $(this).attr('title') + '</h4>';
}
});
});
Update File at Location: app/asset/AppAsset.php
class AppAsset extends AssetBundle {
public $basePath = '@webroot';
public $baseUrl = '@web';
public $css = [
];
public $js = [
'js/ajax-modal-popup.js', //<<<------- Register the script.
];
public $depends = [
'yii\web\YiiAsset',
];
}
Add code chunk to file location: app/views/index.php
<?php
Html::button('Close',
['value' => Url::to(['ticket/close', 'id'=>$model->ticket_id]),
'class' => 'showModalButton btn btn-success']
);
Modal::begin([
'header' => '<h2>Ticket Manager</h2>',
'id' => 'modal',
'size' => 'modal-md',
]);
echo "<div id='modalContent'></div>";
Modal::end();
?>
Add to Controller: app/controllers/ticketController.php
public function actionClose($id) {
$model = $this->findModel($id);
$model->scenario = 'close'; //Applied by Ticket model rules.
if ($model->load(Yii::$app->request->post()) && $model->save()) {
$model->record_void = 1;
$model->save();
return $this->redirect(['index']);
}elseif (Yii::$app->request->isAjax) {
return $this->renderAjax('close', [
'model' => $model
]);
} else {
return $this->render('close', [
'model' => $model
]);
}
}
Your Form File Location: app/views/ticket/close.php
<?php
use yii\helpers\Html;
use yii\widgets\ActiveForm;
/* @var $this yii\web\View */
/* @var $model app\models\Ticket */
/* @var $form ActiveForm */
?>
<div class="ticket-_close">
<h3>Close Ticket</h3>
<?php
$form = ActiveForm::begin(['options' => [
'id' => 'takeModal',
'enableClientValidation' => true,
'enableAjaxValidation' => true,
]]);?>
<?= $form->field($model, 'ticket_id')->textInput(['readonly' => true, 'value' => $model->ticket_id]) ?>
<?= $form->field($model, 'problem') ?>
<?= $form->field($model, 'solution') ?>
<div class="form-group">
<?= Html::submitButton('Submit', ['class' => 'btn btn-primary']) ?>
</div>
<?php ActiveForm::end(); ?>
</div><!-- ticket-_close -->
See below article for additional details.
http://www.yiiframework.com/wiki/806/render-form-in-popup-via-ajax-create-and-update-with-ajax-validation-also-load-any-page-via-ajax-yii-2-0-2-3/