In my case, I had a trailing slash in my IAM identity provider for accounts.google.com, like this:
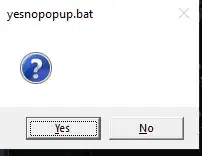
The one with the trailing slash is wrong; the one without the trailing slash works correctly. It's interesting that AWS will fetch the same thumbprint for both of those.
In the AWS IAM console under Accounts > Providers > accounts.google.com, add the key for "Android client for com.example.yourstuff (auto created by Google Service)" as an audience. It looks something like "222222222222-x8x8x8x8x8x8x8x8x8x8x8x8x8x8x8x8.apps.googleusercontent.com" (Then, when you're debugging, go ahead and all the rest of the keys as audience entries; you can go back later and figure out which ones you can remove.)
In the call to GoogleSignInOptions.Builder
, you need a call to #requestIdToken using your web application key under OAuath 2.0 client IDs on the Goole APIs > API Manager > Credentials page:
GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestIdToken("999999whateverxxxx.apps.googleusercontent.com")
.build()
(The token can get cached; if you run your app with the requestIdToken call, then remove the requestIdToken call, and run again, you can still get a result from a call to getIdToken() on the GoogleSignInAccount object.)
The google login code will eventually give you a GoogleSignInAccount
object. Call #getIdToken on that object to get a string (in my case, it's 83 chars) that you're going to put in the login hash:
// pseudocode...
private fun fn(x: GoogleSignInAccount) {
val token = x.idToken // getIdToken if you're still using Java
val logins = HashMap<String, String>()
logins.put("accounts.google.com", token);
credentialsProvider.logins = logins
...
If you don't have the right key listed in IAM > Providers > accounts.google.com, you'll get a NotAuthorizedException(Invalid login token. Incorrect token audience.)
exception.
If you added that extra slash to accounts.google.com/, you'll get a NotAuthorizedException(Token is not from a supported provider of this identity pool.)
If you try to add accounts.google.com/ to the login hash like this (don't do this, fix the IAM identity provider name instead):
logins.put("accounts.google.com/", token);
You'll get a NotAuthorizedException(Invalid login token. Issuer doesn't match providerName)
exception.
If you use the wrong token you'll get a NotAuthorizedException (Invalid login token. Token signature invalid.)
exception.
(I suspect there are many other ways to fail; these are just the one's I've found.)