You can define a servlet in your web.xml as below and then use request.getRequestDispatcher("file").forward(request,response)
, essentially what will happen is that you would be dispatching your request to a servlet whose mapping is /file
and that servlet would point you to your resource /html/file.html
. Please note that even though the element name is jsp-file
but you can point a HTML from it.
<servlet>
<servlet-name>FileServlet</servlet-name>
<jsp-file>/html/file.html</jsp-file>
</servlet>
<servlet-mapping>
<servlet-name>FileServlet</servlet-name>
<url-pattern>/file</url-pattern>
</servlet-mapping>
As an add-on - coming to how URL patterns matches the serlvet mapping present in web.xml file, below are servlet mapping rules in web.xml (sources of this are - Servlet specs and @BalusC answer):
1. Path mapping:
If you want to create a path mapping then start the mapping with /
and end it will /*
. For example:
<servlet-mapping>
<servlet-name>FileServlet</servlet-name>
<url-pattern>/foo/bar/*</url-pattern> <!-- http://localhost:7001/MyTestingApp/foo/bar/index.html would map this servlet -->
</servlet-mapping>
2. Extension mapping:
If you want to create an extension mapping then have servlet mapping *.
. For example:
<servlet-mapping>
<servlet-name>FileServlet</servlet-name>
<url-pattern>*.html</url-pattern> <!-- http://localhost:7001/MyTestingApp/index.html would map this servlet. Also, please note that this servlet mapping would also be selected even if the request is `http://localhost:7001/MyTestingApp/foo/index.html` unless you have another servlet mapping as `/foo/*`. -->
</servlet-mapping>
3. Default servlet mapping:
Suppose you want to define that if a mapping doesn't match any of the servelt mapping then it should be mapped to the default servlet then have servlet mapping as /
. For example:
<servlet-mapping>
<servlet-name>FileServlet</servlet-name>
<url-pattern>/</url-pattern> <!-- Suppose you have mapping defined as in above 2 example as well, and request comes for `http://localhost:7001/MyTestingApp/catalog/index.jsp` then it would mapped with servlet -->
</servlet-mapping>
4. Exact match mapping:
Suppose you want to define exact match mapping then do not use any wild card character or something, and define the exact match, like /catalog
. For example:
<servlet-mapping>
<servlet-name>FileServlet</servlet-name>
<url-pattern>/catalog</url-pattern> <!-- Only requests with http://localhost:7001/MyTestingApp/catalog will match this servlet -->
</servlet-mapping>
5. Application context root mapping:
The empty string ""
is a special URL pattern that exactly maps to the
application's context root. i.e., requests of the form http://localhost:7001/MyTestingApp/
.
<servlet-mapping>
<servlet-name>FileServlet</servlet-name>
<url-pattern></url-pattern> <!-- Only requests with http://localhost:7001/MyTestingApp/ will match this servlet Please note that if request is http://localhost:7001/MyTestingApp/abc then it will not match this mapping -->
</servlet-mapping>
6. Match all mapping:
If you want to match all requests to one mapping or override all other servlet mapping then create a mapping as /*
.
<servlet-mapping>
<servlet-name>FileServlet</servlet-name>
<url-pattern>/*</url-pattern> <!-- This will override all mappings including the default servlet mapping -->
</servlet-mapping>
Below is the summary diagram from JMS specification:
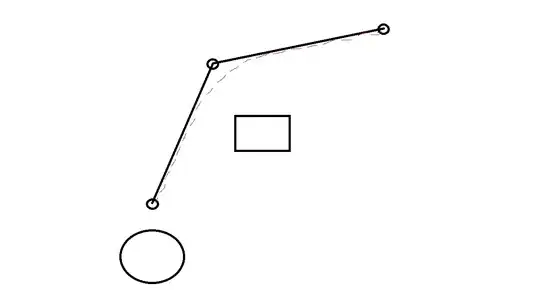