I'm using ItextSharp for applying digital signature to a PDF file. Using below code i'm able to apply signature to a PDF file also able to validate signature, But signatures validation mark is missing from the PDF file's signature box.
Code iTextSharp-(Runtime version = v2.0.50727) (Version = 5.5.10.0)
using Org.BouncyCastle.Pkcs;
using System.IO;
using iTextSharp.text.pdf;
using iTextSharp.text.pdf.security;
public class DigitalSignaturePDF
{
public void DigiSignPdf(string sourceDocument,
string destinationPath,
Stream privateKeyStream,
string keyPassword,
string reason,
string location,
bool isVisibleSignature)
{
Pkcs12Store pk12 = new Pkcs12Store(privateKeyStream, keyPassword.ToCharArray());
privateKeyStream.Dispose();
//then Iterate throught certificate entries to find the private key entry
string alias = null;
foreach (string tAlias in pk12.Aliases)
{
if (pk12.IsKeyEntry(tAlias))
{
alias = tAlias;
break;
}
}
var pk = pk12.GetKey(alias).Key;
// reader and stamper
PdfReader reader = new PdfReader(sourceDocument);
using (FileStream fout = new FileStream(destinationPath, FileMode.Append, FileAccess.Write))
{
using (PdfStamper stamper = PdfStamper.CreateSignature(reader, fout, '\0'))
{
// appearance
PdfSignatureAppearance appearance = stamper.SignatureAppearance;
//appearance.Image = new iTextSharp.text.pdf.PdfImage();
appearance.Reason = reason;
appearance.Location = location;
if (isVisibleSignature)
{
appearance.SetVisibleSignature(new iTextSharp.text.Rectangle(20, 10, 170, 60), reader.NumberOfPages, null);
}
//Get all certificate for validation
X509CertificateEntry[] ce = pk12.GetCertificateChain(alias);
Org.BouncyCastle.X509.X509Certificate[] chain;
chain = new Org.BouncyCastle.X509.X509Certificate[ce.Length];
for (int k = 0; k < ce.Length; ++k)
{
chain[k] = ce[k].Certificate;
}
// digital signature
IExternalSignature es = new PrivateKeySignature(pk, "SHA-256");
MakeSignature.SignDetached(appearance, es, chain, null, null, null, 0, CryptoStandard.CMS);
stamper.Close();
}
}
reader.Close();
reader.Dispose();
}
}
Output file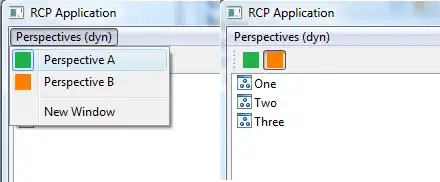
As you can see in above image validation mark is missing from the signature box.
But when i tried with older version of ItextSharp using below code then it is showing green tic mark if signature is valid and a yellow question mark if signature is invalid.
Code iTextSharp-(Runtime version = v1.1.4322) (Version = 3.1.0.0)
public void Sign(string SigReason, string SigContact, string SigLocation, bool visible)
{
PdfReader reader = new PdfReader(this.inputPDF);
PdfStamper st = PdfStamper.CreateSignature(reader, new FileStream(this.outputPDF, FileMode.Create, FileAccess.Write), '\0', null, true);
PdfSignatureAppearance sap = st.SignatureAppearance;
sap.SetCrypto(this.myCert.Akp, this.myCert.Chain, null, PdfSignatureAppearance.SELF_SIGNED);
sap.Reason = SigReason;
sap.Contact = SigContact;
sap.Location = SigLocation;
sap.SetVisibleSignature(new iTextSharp.text.Rectangle(100, 100, 25, 15), 1, null);
st.Close();
}
Output file
In above image a green tic mark is attached with signature box, that is showing that signature is valid.
Can any one have any idea why validation tic is missing when i'm using iTextSharp 5.5.10.0
, what m i missing here.