Using coroutines with Visual Studio 2017 and an MFC solution into which I am using C++/WinRT with its async
type functions, I have the following.
See as well this question and my answer which contains several examples of using coroutines with concurrency
, std:async
and C++/WinRT: C++11 threads to update MFC application windows. SendMessage(), PostMessage() required?
First of all settings in the solution Properties.
- Configuration Properties -> General -> Platform Toolset Visual Studio 2017 (v141)
- C/C++ -> All Options -> Additional Options -> /await
- C/C++ -> All Options -> C++ Language Standard ISO C++17 Standard (/stdc++17)
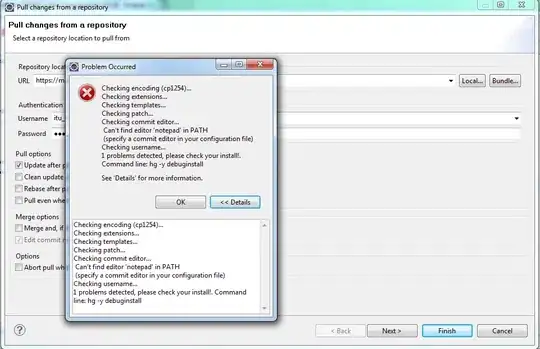
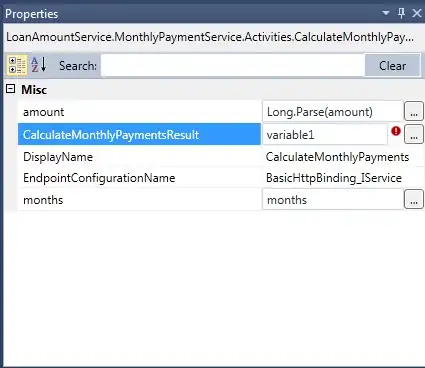
And the source code for the actual function that I am using (C++/WinRT async function with co_await
):
#include <pplawait.h>
#pragma comment(lib, "windowsapp")
#include "winrt/Windows.Foundation.h"
#include "winrt/Windows.Web.Syndication.h"
// . . . other code
// sample function using co_await to retrieve an RSS feed which may take
// a while so we want to start it and then continue when the results are
// received. we are using one of the async functions of C++/WinRT to retrieve
// the RSS feed text. We require a pointer to a CMainFrame object which has
// the function we need to output the text lines to a displayed window.
winrt::Windows::Foundation::IAsyncAction myTaskMain(CMainFrame *p)
{
winrt::Windows::Foundation::Uri uri(L"http://kennykerr.ca/feed");
winrt::Windows::Web::Syndication::SyndicationClient client;
winrt::Windows::Web::Syndication::SyndicationFeed feed = co_await client.RetrieveFeedAsync(uri);
// send the text strings of the RSS feed list to an MFC output window for
// display to the user.
for (winrt::Windows::Web::Syndication::SyndicationItem item : feed.Items())
{
winrt::hstring title = item.Title().Text();
p->SendMessageToOutputWnd(WM_APP, COutputWnd::OutputBuild, (LPARAM)title.c_str()); // print a string to an output window in the output pane.
}
}