Since you just want to show notifications from a firebase service. Look at your manifest file:
<service android:name=".YourFirebaseMessagingService" android:enabled="true" android:exported="true">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>
The service has an intent filter with the com.google.firebase.MESSAGING_EVENT
action, you then just need to implement the service by extending FirebaseMessagingService and overwrite the onMessageReceived
method.
Note that onMessageReceived
is provided for most message types, with the following exceptions:
Notification messages delivered when your app is in the background. In this case, the notification is delivered to the device’s system tray. A user tap on a notification opens the app launcher by default.
Messages with both notification and data payload, both background and foreground. In this case, the notification is delivered to the device’s system tray, and the data payload is delivered in the extras of the intent of your launcher Activity.
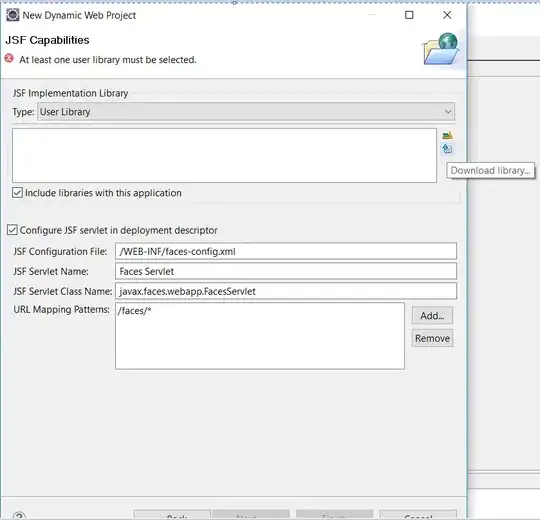
Services run on the main thread unless explicitly told otherwise. This means you can show any notification you'll like, or start a new Activity.
Here's an example of the above:
public class YourFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
if (remoteMessage.getNotification().getBody() != null) {
Log.e("FIREBASE", "Message Notification Body: " + remoteMessage.getNotification().getBody());
//DO WHATEVER YOU NEED HERE TO DISPLAY YOUR MESSAGE OR ACTIVITY
}
}
}
Note that if you wish to wake up your background application, you will need to perform a POST to the following URL:
POST https://fcm.googleapis.com/fcm/send
And the following headers:
Key: Content-Type, Value: application/json
Key: Authorization, Value: key=<your-server-key>
For more information on this, check out this link and this answer.