This example is modified from the official example. Key parts for using colormap in scatter plot are:
x.data
and y.data
are transformed coordinates.
c = np.random.randint(1, 500, size=len(lats))
are values mapping to a color in the colormap corresponding to each point.
Some parts which might be not necessary for you are:
import urllib, os
from netCDF4 import Dataset
import numpy as np
filename, _ = urllib.urlretrieve('http://coastwatch.pfeg.noaa.gov/erddap/tabledap/apdrcArgoAll.nc?longitude,latitude,time&longitude>=0&longitude<=360&latitude>=-90&latitude<=90&time>=2010-01-01&time<=2010-01-08&distinct()')
dset = Dataset(filename)
lats = dset.variables['latitude'][:]
lons = dset.variables['longitude'][:]
dset.close()
os.remove(filename)
c = np.random.randint(1, 500, size=len(lats))
x, y = m(lons,lats)
This part is used for generating an data sample. You might want to replace it with your real data.
from mpl_toolkits.axes_grid1 import make_axes_locatable
divider = make_axes_locatable(ax)
fig.colorbar(pc, cax=divider.append_axes("right", size="5%", pad=0.05))
This is a direct application of bogatron's answer to make the size of colorbar matches the plot. It's your choice of keeping it or not.
import urllib, os
import matplotlib.pyplot as plt
from mpl_toolkits.axes_grid1 import make_axes_locatable
from mpl_toolkits.basemap import Basemap
from netCDF4 import Dataset
import numpy as np
# data downloaded from the form at
# http://coastwatch.pfeg.noaa.gov/erddap/tabledap/apdrcArgoAll.html
filename, _ = urllib.urlretrieve('http://coastwatch.pfeg.noaa.gov/erddap/tabledap/apdrcArgoAll.nc?longitude,latitude,time&longitude>=0&longitude<=360&latitude>=-90&latitude<=90&time>=2010-01-01&time<=2010-01-08&distinct()')
dset = Dataset(filename)
lats = dset.variables['latitude'][:]
lons = dset.variables['longitude'][:]
dset.close()
os.remove(filename)
c = np.random.randint(1, 500, size=len(lats))
# draw map with markers for float locations
fig = plt.figure()
ax = fig.add_subplot(111, axisbg='w', frame_on=True)
fig.set_size_inches(18.5, 10.5)
m = Basemap(lon_0=180, ax=ax)
x, y = m(lons,lats)
pc = m.scatter(x.data, y.data, 20, marker='o', c=c, lw=.25, alpha =0.9, antialiased=True, zorder=3, cmap='summer')
m.fillcontinents(color='#555555')
divider = make_axes_locatable(ax)
fig.colorbar(pc, cax=divider.append_axes("right", size="5%", pad=0.05))
plt.show()
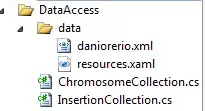