The proper use of a variety of layout managers can greatly increase the ability for a UI to be dynamically sized
Based on the screen shots in your question, I see a BorderLayout
, GridLayout
and possibly a GridBagLayout
as the primary choices
The window "packed"
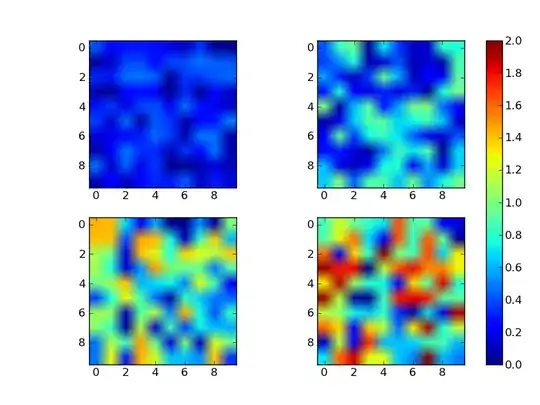
The window "maximised"
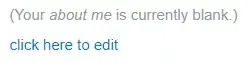
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.GridLayout;
import java.awt.Insets;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextField;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.border.LineBorder;
public class JavaApplication170 {
public static void main(String[] args) {
new JavaApplication170();
}
public JavaApplication170() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new TopPane(), BorderLayout.NORTH);
frame.add(new LeftPane(), BorderLayout.WEST);
frame.add(new JScrollPane(new FieldsPane()));
frame.pack();
//frame.setExtendedState(JFrame.MAXIMIZED_BOTH);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TopPane extends JPanel {
public TopPane() {
setLayout(new GridLayout(1, 0));
add(new JLabel("Some title"));
add(new JButton("One"));
add(new JButton("Two"));
add(new JButton("Three"));
add(new JButton("Four"));
}
}
public class LeftPane extends JPanel {
public LeftPane() {
setLayout(new GridLayout(0, 1));
add(new JButton("One"));
add(new JButton("Two"));
add(new JButton("Three"));
add(new JButton("Four"));
add(new JButton("Five"));
add(new JButton("Six"));
add(new JButton("Seven"));
}
}
public class FieldsPane extends JPanel {
public FieldsPane() {
setLayout(new GridBagLayout());
addField("Field one", 0);
addField("Field two", 1);
addField("Field three", 2);
addField("Field four", 3);
addField("Field five", 4);
addField("Field six", 5);
addField("Field seven", 6);
addField("Field eight", 7);
addField("Field nine", 8);
addField("Field ten", 9);
addField("Field eleven", 10);
addField("Field tweleve", 11);
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridheight = 3;
gbc.gridx = 2;
gbc.gridy = 1;
//gbc.weightx = 1;
add(makeProfileLabel(), gbc);
gbc.gridheight = 1;
gbc.gridy += 3;
add(new JButton("Some button"), gbc);
}
protected void addField(String text, int row) {
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = row;
gbc.insets = new Insets(4, 4, 4, 4);
add(new JLabel(text), gbc);
gbc.gridx++;
add(new JTextField(10), gbc);
}
protected JLabel makeProfileLabel() {
JLabel label = new JLabel();
label.setPreferredSize(new Dimension(50, 50));
label.setBorder(new LineBorder(Color.BLACK));
return label;
}
}
}
Now, this is a relatively simple example intended to demonstrate the concept of using multiple layouts.
For example, I might be tempted to use another GridBagLayout
to layout the "main" layout, which would give me more control over the "some title" label
Layouts can be a bit of a (black magic) art form, but if you start with the "base" requirements, look at the functionality the UI is trying to produce and break those requirements down, it will be easier then trying to dump all the content into a single component