Can't you do a simple interpolation to get the cp value given (1) the V vs cp curve (CSV file) and (2) the velocities from the NetCDF file? Simple interpolations are quite fast in Python (and I guess also in R), and (almost..) certainly faster than implementing something yourself.
If the V vs cp curve from your CSV file is very detailed you could get away with something as simple as a nearest neighbour interpolation, if not there are better alternatives. For example:
import numpy as np
import matplotlib.pylab as pl
from scipy import interpolate
# Given combinations of velocity and cp (from CSV file):
V_csv = np.linspace(0,10,4)
cp_csv = V_csv**2.
# Random velocities in the range {0..10} (from NetCDF file):
V = np.random.random(5)*10
# Create linear, nearest neighbour and quadratic interpolation functions
f_near = interpolate.interp1d(V_csv, cp_csv, kind='nearest')
f_lin = interpolate.interp1d(V_csv, cp_csv, kind='linear')
f_quad = interpolate.interp1d(V_csv, cp_csv, kind='quadratic')
# Interpolate:
cp_near = f_near(V)
cp_lin = f_lin(V)
cp_quad = f_quad(V)
# Visulalization
pl.figure()
pl.plot(np.linspace(0,10,256), np.linspace(0,10,256)**2., label='Real V vs cp curve')
pl.plot(V_csv, cp_csv, '-x', label='Given (discrete) V vs. cp curve')
pl.scatter(V, cp_near, label='nearest')
pl.scatter(V, cp_lin, label='linear')
pl.scatter(V, cp_quad, label='quadratic')
pl.legend(frameon=False, loc='best')
pl.xlabel('V')
pl.ylabel('cp')
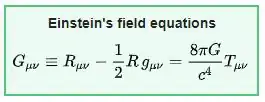