1. Is there any reason why the onChange
attribute would be specifically applied to a group of radio buttons and not to groups of other form elements?
No, there is no reason why it couldn't be applied to other form elements or even the root element of the DOM. All events go from the root to the target (the capture phase) and then back to the root (the bubbling phase). The event handler just has to be written with this in mind. See this SO thread for more info on event bubbling.
2. Why not place all form fields inside a single div or form element and have only one onChange attribute?
This is usually true when it comes to vanilla JS. However, React implements its own event system, and the way that they seem to advise is to create an event listener and then link each input to it.
<form onSubmit={handleSubmit}>
<input type="text" onChange={handleChange} />
<input type="text" onChange={handleChange} />
<input type="text" onChange={handleChange} />
</form>
The handleChange
function will use event.target.value
whether it uses event bubbling or not, but React seems to prefer that you explicitly attach the function to each input element.
If you don't, it's common to run into warnings when you try to set default values:
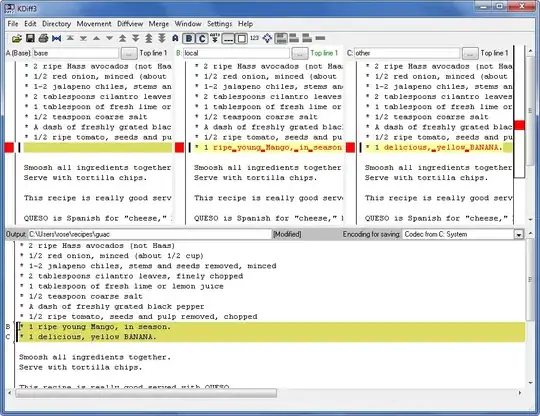
(default values have also been a common source of confusion, more info here)
It is just a warning. This warning has been the subject of a large discussion on the Github repo, more discussion on that below.
3. Is this specific to ReactJS, or can something similar be done in basic JavaScript?
As mentioned, this is not specific to React, it is in fact more specific to JS. React seems to be agnostic about it. It would seem that they prefer you add the onChange
reference to each input element and not use bubbling in this way.
What is the right way?
I don't know. I think it comes down to what you prefer, or what the situation calls for.
In the large discussion on the Github repo, most people there run into the warnings because using event bubbling is usually the way that is encouraged as 'best practice' in vanilla JS. However, there are also voices that say:
This is my opinion, but I'd say it's the opposite, it's a bad idea. It relies on bubbling outside of the React hierarchy.
link to comment
So depending on who you ask, using event bubbling may be considered against the trend of React thinking. It will depend on how you choose to architect your app.
Its important to note that there is no real issue with it, everything works. Whether you use event bubbling or not. The main gripe, in the issue, seems to come down to the fact that the console becomes crowded with warnings and there is no elegant way to turn them off. The best way appears to be to just assign an empty function to the input elements.