See this question. The answer by Jobi Joy will let you present the 2D list but Binding won't work so you can't edit your values.
To be able to bind the values you can use a helper class like this
public static class BindableListHelper
{
public static List<List<Ref<T>>> GetBindable2DList<T>(List<List<T>> list)
{
List<List<Ref<T>>> refInts = new List<List<Ref<T>>>();
for (int i = 0; i < list.Count; i++)
{
refInts.Add(new List<Ref<T>>());
for (int j = 0; j < list[i].Count; j++)
{
int a = i;
int b = j;
refInts[i].Add(new Ref<T>(() => list[a][b], z => { list[a][b] = z; }));
}
}
return refInts;
}
}
This method uses this Ref class
public class Ref<T>
{
private readonly Func<T> getter;
private readonly Action<T> setter;
public Ref(Func<T> getter, Action<T> setter)
{
this.getter = getter;
this.setter = setter;
}
public T Value { get { return getter(); } set { setter(value); } }
}
Then you can set ItemsSource for the ItemsControl with
itemsControl.ItemsSource = BindableListHelper.GetBindable2DList<bool>(Checkboxes);
and the editing should work
Using the same code as Jobi Joy from the question I linked, you can change the Button in DataTemplate_Level2 to a CheckBox and bind IsChecked for it to Value instead (since it will point to the Ref class otherwise)
<Window.Resources>
<DataTemplate x:Key="DataTemplate_Level2">
<CheckBox IsChecked="{Binding Path=Value}" Height="15" Width="15" Margin="2"/>
</DataTemplate>
<DataTemplate x:Key="DataTemplate_Level1">
<ItemsControl ItemsSource="{Binding}" ItemTemplate="{DynamicResource DataTemplate_Level2}">
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<StackPanel Orientation="Horizontal"/>
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
</ItemsControl>
</DataTemplate>
</Window.Resources>
<StackPanel>
<Border HorizontalAlignment="Left" BorderBrush="Black" BorderThickness="2">
<ItemsControl x:Name="itemsControl" ItemTemplate="{DynamicResource DataTemplate_Level1}"/>
</Border>
</StackPanel>
Without setting the Content property for the CheckBox it'll look something like this
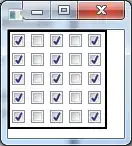