I prepared an MCVE to demonstrate the options I suggested in my comments.
Changing style of QLabel
is rather simple and straight forward.
Using the QStylePainter
doesn't quite match what I actually expected. I left in the sample code what I got so far. (May be, somebody could leave a helpful hint.) I will investigate in this topic later and edit this answer as soon as I got some satisfying progress on this topic.
The sample code:
#include <QtWidgets>
class TitleBar: public QWidget {
private:
QStyleOptionTitleBar _option;
//QString _text;
public:
explicit TitleBar(
const QString &text = QString(), QWidget *pQParent = nullptr);
protected:
virtual QSize sizeHint() const;
virtual void resizeEvent(QResizeEvent *pQEvent);
virtual void paintEvent(QPaintEvent *pQEvent);
};
int main(int argc, char **argv)
{
qDebug() << "Qt Version: " << QT_VERSION_STR;
// main application
#undef qApp // undef macro qApp out of the way
QApplication qApp(argc, argv);
// setup of GUI
QWidget qWin;
QVBoxLayout qBox;
// a boring label
QLabel qLbl1(QString::fromUtf8("Rather boring"));
qLbl1.setAlignment(Qt::AlignCenter);
qBox.addWidget(&qLbl1);
// a label with frame
QLabel qLbl2(QString::fromUtf8("Label with Frame"));
qLbl2.setFrameStyle(QLabel::Panel | QLabel::Raised);
qLbl2.setLineWidth(2);
qLbl2.setAlignment(Qt::AlignCenter);
qBox.addWidget(&qLbl2);
// a label with rich-text
QLabel qLbl3(
QString::fromUtf8(
"<body bgcolor='#28f'>" // doesn't have the effect
"<font size='+2' color='#f8e' face='Old English Text MT'>"
"Label <i>with</i> <b>Rich-Text</b>"
"</font>"
"</body>")); // background is set by style-sheet instead
qLbl3.setTextFormat(Qt::RichText); // might be auto-detected...
qLbl3.setStyleSheet("QLabel { background-color: #28f; }");
qLbl3.setAlignment(Qt::AlignCenter);
qLbl3.show();
qBox.addWidget(&qLbl3);
// a home-brew title bar
TitleBar qTitle("A Home-Brew Title Bar");
qBox.addWidget(&qTitle);
// finish setup of GUI
qWin.setLayout(&qBox);
qWin.show();
// run application
return qApp.exec();
}
TitleBar::TitleBar(const QString &text, QWidget *pQParent):
QWidget(pQParent)
{
_option.initFrom(this);
_option.titleBarFlags = Qt::Window;
_option.text = text;
}
QSize TitleBar::sizeHint() const
{
#if 0 // does not provide the expected result
return _option.rect.size();
#elif 0 // does not provide the expected result
return style()->proxy()->subControlRect(QStyle::CC_TitleBar,
&_option, QStyle::SC_TitleBarLabel).size();
#else
return QSize(0,
style()->proxy()->pixelMetric(QStyle::PM_TitleBarHeight,
&_option, this));
#endif // 0
}
void TitleBar::resizeEvent(QResizeEvent *pQEvent)
{
_option.rect = QRect(_option.rect.topLeft(), pQEvent->size());
}
void TitleBar::paintEvent(QPaintEvent *pQEvent)
{
QPainter qPainter(this);
style()->proxy()->drawComplexControl(QStyle::CC_TitleBar,
&_option, &qPainter, this);
}
I compiled and tested it in VS2013 on Windows 10 (64 bit). Below a snapshot:
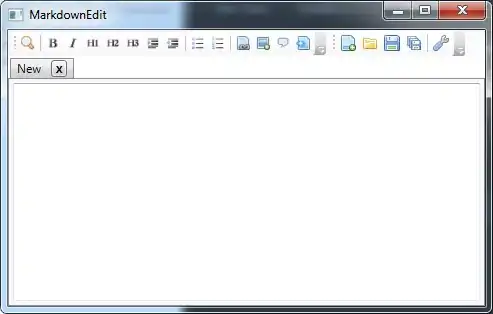