Assuming your sheets are as follows:
Sheet1
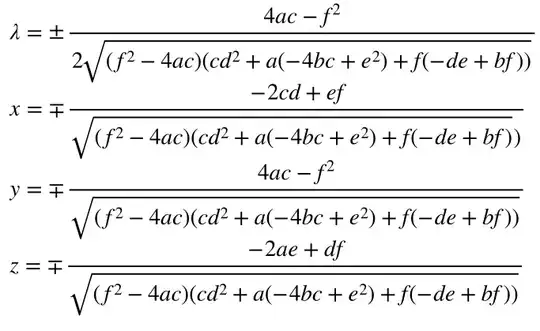
Sheet2
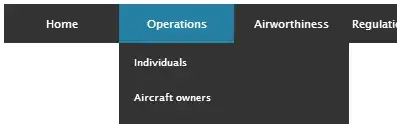
In Cell A2
of Sheet3
enter the formula
=IFERROR(INDEX(Sheet1!$A$2:$A$8,MATCH(SMALL(IF(COUNTIF(Sheet1!$C$2:$C$10,Sheet2!$C$2:$C$10),Sheet2!$C$2:$C$10),ROW(1:1)),Sheet1!$C$2:$C$8,0)),"")
and in Cell B2
of Sheet3
enter the following formula
=IFERROR(INDEX(Sheet2!$B$2:$B$8,MATCH(SMALL(IF(COUNTIF(Sheet1!$C$2:$C$10,Sheet2!$C$2:$C$10),Sheet2!$C$2:$C$10),ROW(1:1)),Sheet1!$C$2:$C$8,0)),"")
Both the above formula are array formula so commit it by pressing Ctrl+Shift+Enter. Drag/Copy down as required. See image for reference.

-----------------------------------------------------------------------------------------------------------------------
If you also want to display third column of first two sheets in Sheet3
(which is ID
in my sample sheet) then enter following formula in Cell A2
=IFERROR(SMALL(IF(COUNTIF(Sheet1!$C$2:$C$10,Sheet2!$C$2:$C$10),Sheet2!$C$2:$C$10),ROW(1:1)),"")
This is also an array formula. In Cell B2
enter
=IFERROR(INDEX(Sheet1!$A$2:$A$8,MATCH(A2,Sheet1!$C$2:$C$8,0)),"")
And in Cell C2
enter
=IFERROR(INDEX(Sheet2!$B$2:$B$8,MATCH(A2,Sheet2!$C$2:$C$8,0)),"")
Drag/Copy down as required. See image below.
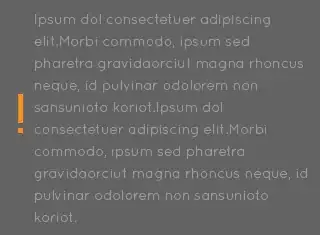
Got this from @ScottCraner's answer here.
There's another way of achieving this without using using formula and VBA. See if this helps.