You might have success with Sergiy Medvynskyy's answer on this question, or at least use it in some way;
JLabel label = new JLabel("MESSAGE");
label.setFont(new Font("Arial", Font.BOLD, 18));
JOptionPane.showMessageDialog(null,label,"ERROR",JOptionPane.WARNING_MESSAGE);
Since JOptionPane
accepts a JLabel as parameter, you could experiment a bit by trying to resize that one, and then just passing it on.
Edit:
Assuming you are using Swing, this code should work.
(You might have to experiment a bit to reach your desired size/layout)
TextField tf = new TextField();
tf.setFont(new Font("Arial", Font.BOLD, 20));
JPanel jp = new JPanel(new BorderLayout(0, 0));
JLabel jl = new JLabel("Example Prompt Message!");
jl.setFont(new Font("Arial", Font.BOLD, 16));
jp.add(jl, BorderLayout.NORTH);
jp.add(tf, BorderLayout.SOUTH);
JOptionPane.showConfirmDialog(null, jp);
Result should be something similiar to this. (You can change the dialogue type too of course, though showInputDialog
probably won't work, due to it already having a default input line.)
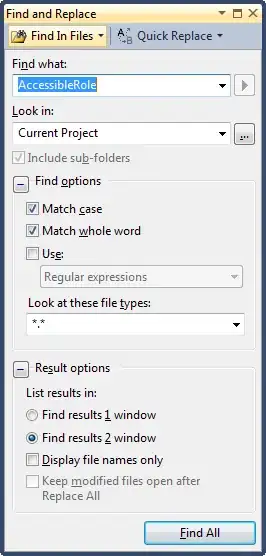