There are a lot of ways to generate random numbers between two values and a specific mean. Python has built in methods for some of these ways, but when I say there are a lot of ways, I mean it.
The simplest way would be to use a triangular distribution. random.triangular(low, high, mode)
will produce a number from a distribution between low and high, with a specified mode. This might be good enough, but if you really want a mean, you can use the following function:
def triangular_mean(low, high, mean):
mode = 3 * mean - low - high
return random.triangular(low, high, mode)
If you wanted to get more complex, you could use a beta distribution by calling random.beta(alpha,beta)
. These are really flexible and very weird; this image from Wikipedia highlights how strange they can be.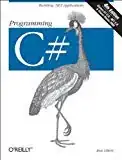
The mean of a beta distribution is alpha/(alpha+beta)
, and the results are always between zero and one, so to scale it up to your use case, let's wrap it up in this function:
def beta_mean(low, high, mean, alpha):
offset = low
scale = high - low
true_mean = (mean - offset)/scale
beta = (alpha/true_mean) - alpha
return offset + scale * random.beta(alpha, beta)
In the above function, alpha will change the shape of the distribution without changing the mean; it will change the median, the mode, the variance, and other properties of the distribution.
There are other distributions you could wrap in functions to fit your use case, but I'm going to guess that the above two will fit whatever use case you would like.
These will also produce floating point numbers, so if you want integers you'll have to cast them to integers, either by editing the functions or casting them explicitly after calling them.