I have one example in my pj, it's in Swift but I guess you can convert it fine, tweak the code as you needed, 55 is the length of each line
To create the corner, maybe this will do, scanRectView
is the interest rect:
func createFrame() -> CAShapeLayer {
let height: CGFloat = self.scanRectView.frame.size.height
let width: CGFloat = self.scanRectView.frame.size.width
let path = UIBezierPath()
path.move(to: CGPoint(x: 5, y: 50))
path.addLine(to: CGPoint(x: 5, y: 5))
path.addLine(to: CGPoint(x: 50, y: 5))
path.move(to: CGPoint(x: height - 55, y: 5))
path.addLine(to: CGPoint(x: height - 5, y: 5))
path.addLine(to: CGPoint(x: height - 5, y: 55))
path.move(to: CGPoint(x: 5, y: width - 55))
path.addLine(to: CGPoint(x: 5, y: width - 5))
path.addLine(to: CGPoint(x: 55, y: width - 5))
path.move(to: CGPoint(x: width - 55, y: height - 5))
path.addLine(to: CGPoint(x: width - 5, y: height - 5))
path.addLine(to: CGPoint(x: width - 5, y: height - 55))
let shape = CAShapeLayer()
shape.path = path.cgPath
shape.strokeColor = UIColor.white.cgColor
shape.lineWidth = 5
shape.fillColor = UIColor.clear.cgColor
return shape
}
Objective C code :
CGFloat height = baseScannerView.frame.size.height+4;
CGFloat width = baseScannerView.frame.size.width+4;
UIBezierPath* path = [UIBezierPath bezierPath];
[path moveToPoint:CGPointMake(1, 25)];
[path addLineToPoint:CGPointMake(1, 1)];
[path addLineToPoint:CGPointMake(25, 1)];
[path moveToPoint:CGPointMake(width - 30, 1)];
[path addLineToPoint:CGPointMake(width - 5, 1)];
[path addLineToPoint:CGPointMake(width - 5, 25)];
[path moveToPoint:CGPointMake(1, height - 30)];
[path addLineToPoint:CGPointMake(1, height - 5)];
[path addLineToPoint:CGPointMake(25, height - 5)];
[path moveToPoint:CGPointMake(width - 30, height - 5)];
[path addLineToPoint:CGPointMake(width - 5, height - 5)];
[path addLineToPoint:CGPointMake(width - 5, height - 30)];
CAShapeLayer *pathLayer = [CAShapeLayer layer];
pathLayer.path = path.CGPath;
pathLayer.strokeColor = [[UIColor redColor] CGColor];
pathLayer.lineWidth = 5.0f;
pathLayer.fillColor = nil;
[self.scanRectView.layer addSublayer:pathLayer];
After create, add it like self.scanRectView.layer.addSublayer(self.createFrame())
in viewWillAppear
or viewDidLayoutSubView
Result:
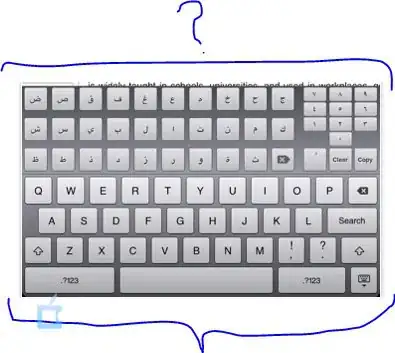