You can try to use the following code to count the number of documents in your collection.
client = new DocumentClient(new Uri(EndpointUri), PrimaryKey);
IQueryable<int> total = client.CreateDocumentQuery<int>(UriFactory.CreateDocumentCollectionUri("testdb", "testcoll"), "SELECT Value count(1) FROM c", new FeedOptions() { EnableCrossPartitionQuery = true });
Console.WriteLine("total: " + total.AsEnumerable().FirstOrDefault());
Query result:
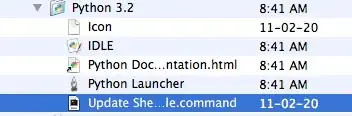
If you capture the request (using fiddler etc), you will find that the client sdk send the same query {"query":"SELECT VALUE [{\"item\": Count(1)}]\r\nFROM root"}
to the server when you execute your code docDbClient.CreateDocumentQuery<TResult>().Count()
.