If you create a Reactive Form you can dynamically change the validation
component
this.questionForm = fb.group({
questions: fb.array(this.questions.map(this.createQuestionControl(fb)))
});
createQuestionControl(fb: FormBuilder) {
return (question, index) => {
const checkbox = question.selected
const answerbox = question.selected ? ['', [Validators.required, Validators.minLength(4)]] : ''
return fb.group({question: checkbox, answer: answerbox, questionNumber: index + 1});
}
}
changeValidator(selected, index) {
const answerbox = this.questionForm.get('questions.' + index).get('answer')
const validators = selected ? [Validators.required, Validators.minLength(4)] : null
answerbox.setValidators(validators);
answerbox.updateValueAndValidity();
}
The createQuestionControl()
method will change each question into a control as below which the form builder can turn into a group with a question and an answer
{ question: true, answer: ['', [Validators.required, Validators.minLength(4)]], index: 4 }
The changeValidator()
method will add or remove validators on the answer if the question is changed (note: do not forget updateValueAndValidity
)
template
<form [formGroup]="questionForm" (ngSubmit)="submit(questionForm)">
<div formArrayName="questions">
<div *ngFor="let question of questionForm.get('questions').controls | orderBySelected; let i = index;" [formGroupName]="i">
<!--{{questionForm.get('questions.' + i + '.questionNumber').value}}-->
{{questions[questionForm.get('questions.' + i + '.questionNumber').value - 1]['EN']}}
<input type="checkbox" formControlName="question" (ngModelChange)="changeValidator($event, i)"/>
<input type="text" formControlName="answer" />
<em *ngIf="questionForm.get('questions.' + i + '.answer').invalid">Minimum length 4</em>
</div>
</div>
<button type="submit" [disabled]="questionForm.invalid">Submit</button>
</form>
Following a clarification in the comments:
Maximum of 5 can be checked at a given time
I have updated the array to have cross field validation of no more than 3 (easier to test you can change it to 5)
export function max3Selected(formArray) {
let totalSelected = formArray.controls.reduce((selectedControls, control) =>
{
if (control.get('question').value) {
selectedControls++
}
return selectedControls;
}, 0)
return totalSelected > 3 ? { moreThanThreeSelected: true } : null;
}
and you would change the fb.array
to include the validator function
fb.array(this.questions.map(this.createQuestionControl(fb)), max3Selected)
Screenshot of result
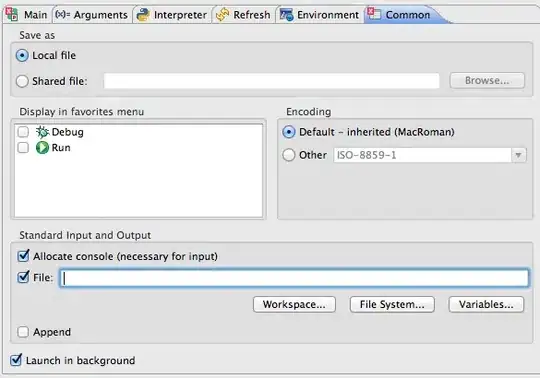
Live plunker example