You can fix the order of magnitude to show on the axis as shown in this question. The idea is to subclass the usual ScalarFormatter
and fix the order of magnitude to show. Then setting the useOffset
to False will prevent showing some offset, but still shows the factor.
Thwe format "%1.1f"
will show only one decimal place. Finally using a MaxNLocator
allows to set the maximum number of ticks on the axes.
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.ticker
class OOMFormatter(matplotlib.ticker.ScalarFormatter):
def __init__(self, order=0, fformat="%1.1f", offset=False, mathText=True):
self.oom = order
self.fformat = fformat
matplotlib.ticker.ScalarFormatter.__init__(self,useOffset=offset,useMathText=mathText)
def _set_orderOfMagnitude(self, nothing):
self.orderOfMagnitude = self.oom
def _set_format(self, vmin, vmax):
self.format = self.fformat
if self._useMathText:
self.format = '$%s$' % self.format
x = [0.6e-8+14.41249698, 3.4e-8+14.41249698]
y = [-7.7e-11-1.110934954e-2, -0.8e-11-1.110934954e-2]
fig, ax = plt.subplots()
ax.plot(x,y)
y_formatter = OOMFormatter(-2, "%1.1f")
ax.yaxis.set_major_formatter(y_formatter)
x_formatter = OOMFormatter(1, "%1.1f")
ax.xaxis.set_major_formatter(x_formatter)
ax.xaxis.set_major_locator(matplotlib.ticker.MaxNLocator(2))
ax.yaxis.set_major_locator(matplotlib.ticker.MaxNLocator(2))
plt.show()
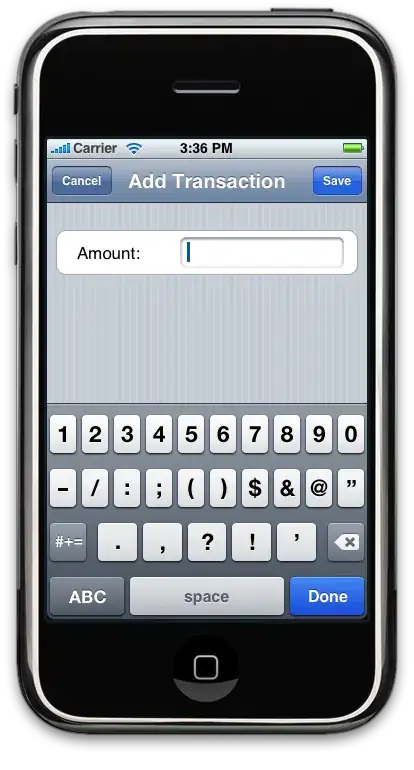
Keep in mind that this plot is inaccurate and you shouldn't use it in any kind of report you hand to other people as they wouldn't know how to interprete it.