If you end up doing a lot with math and formulas you may wish to consider using LaTex which can be converted to a pdf.
I don't think what you are asking is doable with the drawString method but reportlab offers another method that allows it.
This article should be of great help to you: https://www.blog.pythonlibrary.org/2018/02/06/reportlab-101-the-textobject/
Adapting directly from the article gives us this code:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
def apply_scripting(textobject, text, rise):
textobject.setFont("Helvetica-Oblique", 8)
textobject.setRise(rise)
textobject.textOut(text)
textobject.setFont("Helvetica-Oblique", 12)
textobject.setRise(0)
def main():
canvas_obj = canvas.Canvas("textobj_rising.pdf",
pagesize=letter)
# Create textobject
textobject = canvas_obj.beginText()
textobject.setFont("Helvetica-Oblique", 12)
# Set text location (x, y)
textobject.setTextOrigin(10, 730)
textobject.textOut('37')
apply_scripting(textobject, '%', 4)
canvas_obj.drawText(textobject)
canvas_obj.save()
if __name__ == '__main__':
main()
Which creates a pdf like this:
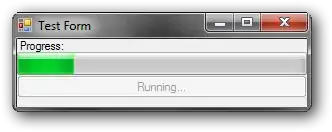
The advantage of this over drawString twice is you don't need to figure out the coordinates for where to place the %
symbol.