I know it's too late to post this answer but for others, it will help
OpenStreetMap(OSM) does not offer any aerial imagery layer(satellite view). But using MAPBOX we can achieve satellite view in OSM.
To do this use the following code snippet:
Add this meta data in AndroidManifest
<meta-data
android:name="MAPBOX_MAPID"
android:value="satellite-streets-v11"/>
<meta-data
android:name="MAPBOX_ACCESS_TOKEN"
android:value="PUT_YOUR_MAPBOX_ACCESS_TOKEN"/>
Create MapBoxTileSourceFixed.java class as follow
//waiting for osmdroid #1718 to be fixed:
class MapBoxTileSourceFixed extends MapBoxTileSource {
MapBoxTileSourceFixed(String name, int zoomMinLevel, int zoomMaxLevel, int tileSizePixels) {
super(name, zoomMinLevel, zoomMaxLevel, tileSizePixels, "");
}
@Override public String getTileURLString(final long pMapTileIndex) {
StringBuilder url = new StringBuilder("https://api.mapbox.com/styles/v1/mapbox/");
url.append(getMapBoxMapId());
url.append("/tiles/");
url.append(MapTileIndex.getZoom(pMapTileIndex));
url.append("/");
url.append(MapTileIndex.getX(pMapTileIndex));
url.append("/");
url.append(MapTileIndex.getY(pMapTileIndex));
//url.append("@2x"); //for high-res
url.append("?access_token=").append(getAccessToken());
String res = url.toString();
return res;
}
}
And finally set MapBoxTileSource in mapview as follow
OnlineTileSourceBase MAPBOXSATELLITELABELLED = new MapBoxTileSourceFixed("MapBoxSatelliteLabelled", 1, 19, 256);
((MapBoxTileSource) MAPBOXSATELLITELABELLED).retrieveAccessToken(this);
((MapBoxTileSource) MAPBOXSATELLITELABELLED).retrieveMapBoxMapId(this);
TileSourceFactory.addTileSource(MAPBOXSATELLITELABELLED);
map.setTileSource(MAPBOXSATELLITELABELLED);
map.getOverlayManager().getTilesOverlay().setColorFilter(null);
Output:
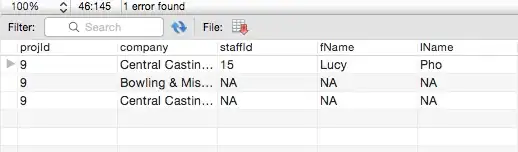
see original repository here
Enjoy coding :)