That can be easily achieved with Transitions API.
With Transitions API you do not take care of writing animations, you just tell what you want the end values be and Transitions API would take care of constructing animations.
Having this xml as content view (a view in the center of the screen):
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/root"
android:layout_width="match_parent"
android:layout_height="match_parent">
<View
android:id="@+id/view"
android:layout_width="120dp"
android:layout_height="80dp"
android:layout_gravity="center"
android:background="@color/colorAccent" />
</FrameLayout>
In activity:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.item)
val root = findViewById(R.id.root) as ViewGroup
val view = findViewById(R.id.view)
view.setOnClickListener {
// After this line Transitions API would start counting the delta
// and will take care of creating animations for each view in `root`
TransitionManager.beginDelayedTransition(root)
// By default AutoTransition would be applied,
// but you can provide your transition with the second parameter
// val transition = AutoTransition()
// transition.duration = 2000
// TransitionManager.beginDelayedTransition(root, transition)
// We are changing size of the view to match parent
val params = view.layoutParams
params.height = ViewGroup.LayoutParams.MATCH_PARENT
params.width = ViewGroup.LayoutParams.MATCH_PARENT
view.requestLayout()
}
}
Here's the output:
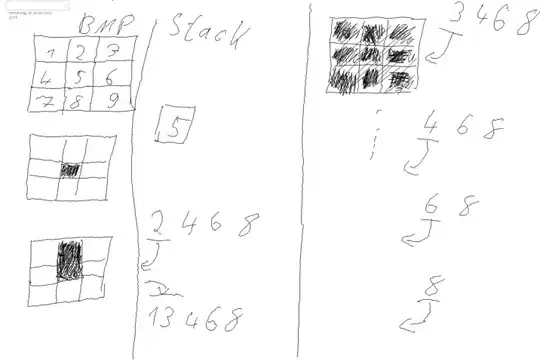
Platform's Transitions API (android.transition.TransitionManager
) is available from API 19, but support libraries backport the functionality upto API 14 (android.support.transition.TransitionManager
).