With a meager amount of information from your question, hopefully I understand the problem correctly.
I have created an example that has a UIViewController
with a UILabel
and a UIButton
.
Whenever the button is pressed, the screen will display an action sheet menu with three buttons (Copy
, Paste
, View Memory
).
And when the Copy
button in the action sheet is pressed, we will copy the text of the label.
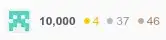
This is the code:
Note:
UILabel
named displayResultLabel
.
UIButton
's action named showActionSheetButtonAction
.
class ViewController: UIViewController {
@IBOutlet weak var displayResultLabel: UILabel!
@IBAction func showActionSheetButtonAction(_ sender: UIButton) {
let actionSheetController = UIAlertController(title: nil, message: nil, preferredStyle: .actionSheet)
actionSheetController.addAction(
UIAlertAction(title: "Copy", style: .default, handler: { [weak self] _ in
guard let strongSelf = self else { return }
UIPasteboard.general.string = strongSelf.displayResultLabel.text
})
)
actionSheetController.addAction(
UIAlertAction(title: "Paste", style: .default, handler: { _ in
// Where to handle when the Paste button is pressed
})
)
actionSheetController.addAction(
UIAlertAction(title: "View Memory", style: .default, handler: { _ in
// Where to handle when the View Memory button is pressed
})
)
actionSheetController.addAction(UIAlertAction(title: "Cancel", style: .cancel, handler: nil))
present(actionSheetController, animated: true, completion: nil)
}
}