You could make a fill in the blanks type format using constant text inside EditText. Do this by creating an EditText in XML.
<EditText
android:id="@+id/editText"
android:layout_height="wrap_content"
android:layout_width="match_parent"/>
In your Java class add the following code:
final EditText edittext = (EditText) findViewById(R.id.editText);
edittext.setText("Something ");
Selection.setSelection(edittext.getText(), edittext.getText().length());
edittext.addTextChangedListener(new TextWatcher() {
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
// TODO Auto-generated method stub
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
// TODO Auto-generated method stub
}
@Override
public void afterTextChanged(Editable s) { if(!s.toString().contains("Something ")){
edittext.setText("Something ");
Selection.setSelection(edittext.getText(), edittext.getText().length());
}
}
});
This is output of the code. Here the string Something is static and cannot be removed.
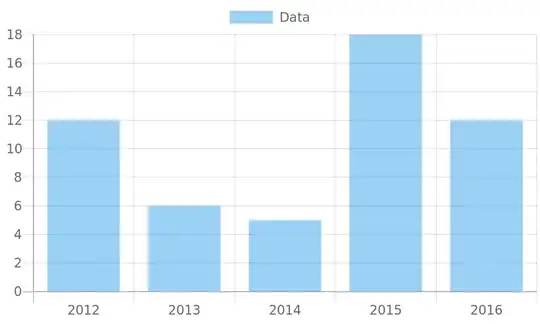
You could hide the underline in Edittext using android:background="@android:color/transparent"
. I would also recommend you to disable enter in the EditText by using the code:
<EditText
android:id="@+id/editText"
android:layout_height="wrap_content"
android:layout_width="match_parent"
android:maxLines="1" <----- add this
android:inputType="text"/> <------ and this to disable enter in edittext