Other Answers are correct. You are reusing your second ArrayList
, just not in the fashion you intended.
You need to understand (a) references to objects, and (b) ArrayList is an object that is a container of references to other objects.
Let's look at the state during the execution of your code.
First instantiate an ArrayList
with the default of ten slots. Will hold references to other ArrayList
instances that hold references to String
objects.
ArrayList< ArrayList< String > > arrayListContainer = new ArrayList<>();
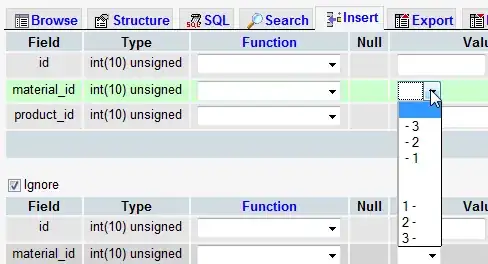
ArrayList< String > temporaryArrayList = new ArrayList<>() ;
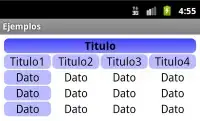
Instantiate String
objects, adding a reference to each in a slot in our second list. Remember the list contains a reference to the String
object, not the object itself.
temporaryArrayList.add( "I" ) ;
temporaryArrayList.add( "you" ) ;
temporaryArrayList.add( "he" ) ;
temporaryArrayList.add( "she" ) ;
temporaryArrayList.add( "we" ) ;
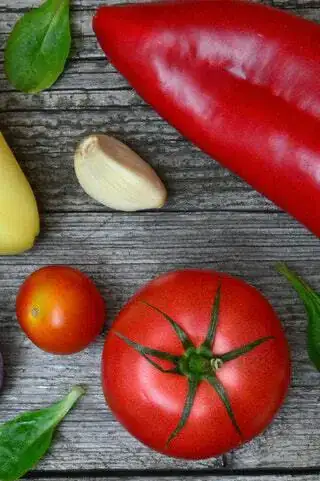
Add a reference to the second list to the first slot of the first list.
arrayListContainer.add(temporaryArrayList) ;

Remove all the references to the String
objects with pronouns by calling clear()
. Those String
objects actually exist in memory for a while, becoming candidates for garbage collection since they are not referenced by any other objects. So we show them as gone in this diagram, but actually they may be floating around in memory for a while longer.
temporaryArrayList.clear() ;
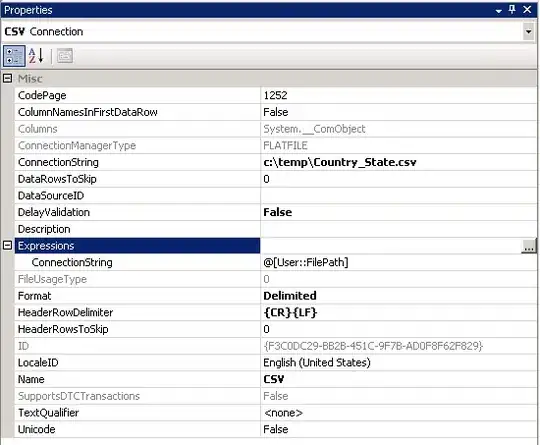
Instantiate some new String
objects. Put a reference to each in the slots of our second list.
temporaryArrayList.add( "Rahim" ) ;
temporaryArrayList.add( "jon" ) ;
temporaryArrayList.add( "don" ) ;
temporaryArrayList.add( "shaila" ) ;

Here is where your misunderstanding appears. You apparently wanted to keep the pronouns and add some proper names alongside them. But the first list does not hold references to String
objects, it holds a reference to an ArrayList
of String
objects. So we end up two references to the same ArrayList
object containing references to proper name strings. The old pronoun strings may or may not be still floating around in memory, but we can no longer reach them so we think of them as gone.
arrayListContainer.add( temporaryArrayList ) ;
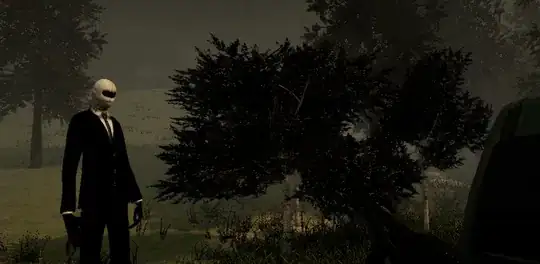
Next you clear the one-and-only list of strings. So now the first ArrayList
hold two references to the same now-empty second ArrayList
.
temporaryArrayList.clear() ;
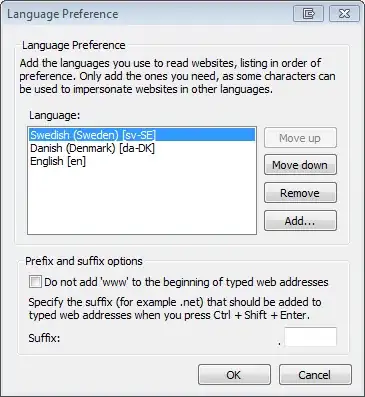
You end up with one list of two elements where each element is pointing to the same empty "temp" list.
If you wanted to accumulate the strings in the first list, redefine that first list as an ArrayList
of String
rather than of ArrayList<String>
. So, instead of this:
ArrayList< ArrayList< String > > arrayListContainer = new ArrayList<>() ;
ArrayList< String > temporaryArrayList = new ArrayList<>() ;
…
arrayListContainer.add( temporaryArrayList ) ;
…this, changing add
to addAll
:
ArrayList< String > arrayListContainer = new ArrayList<>() ;
ArrayList< String > temporaryArrayList = new ArrayList<>() ;
…
arrayListContainer.addAll( temporaryArrayList ) ; // Call `addAll` to add the elements of one collection to another collection.
Tip: Use interfaces where possible.
Generally best to specify more generic interfaces rather than concrete classes, so you can more easily switch out concrete classes; so you could use List
: List< List<String> >
.
List<List<String>> listContainer = new ArrayList<>();