AFAIK, for using App Service Authentication / Authorization, your C# Web API need to be deployed to azure. App Service Authentication / Authorization (Easy Auth) is a feature of Azure App Service and is implemented as a native IIS module that runs in the same sandbox as your azure application. For more details, you could refer to Architecture of Azure App Service Authentication / Authorization.
Based on your scenario, you could refer to the following approaches:
Use App Service Authentication / Authorization (Easy Auth)
configure your App Service application to use Microsoft Account login
Access https://{your-appname}.azurewebsites.net/.auth/login/microsoftaccount
via the browser to ensure that your have successfully set up your Web API and only the users authenticated by Microsoft account could access your Web API
For your front-end web app, you could leverage the JavaScript client library for Azure Mobile Apps for logging and retrieve the authenticationToken
and userId
, then you could set the x-zumo-auth
request header with the value authenticationToken
as the token for accessing your Web API as follows:
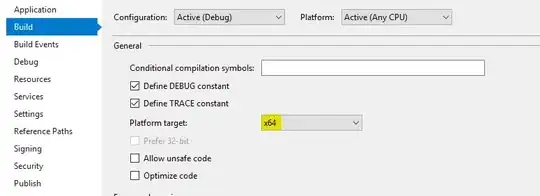
For more details about how to authenticate the user, you could refer to How to: Authenticate users.
Note: For your local SPA, you need to configure the CORS setting and add ALLOWED EXTERNAL REDIRECT URLS under "SETTINGS > Authentication / Authorization" of your azure web app. For more details, you could refer to this issue.
Set up the authentication in your Web API
You could set up your OAuth 2.0 Bearer authentication, with AD v2.0 endpoint you could protect your Web API with both person MSA and work or school accounts. For how to build your Web API, you could refer to Secure an MVC web API.
For your front-end web app, you could leverage MSAL.js library for logging and retrieve the access_token, and use the token for calling your Web API HTTP bearer request.
UPDATE:
I used the TodoListService project from AppModelv2-WebAPI-DotNet, then use the following html for my client as follows:
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
<meta charset="utf-8" />
<!-- IE support: add promises polyfill before msal.js -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/bluebird/3.3.4/bluebird.min.js" class="pre"></script>
<script src="https://secure.aadcdn.microsoftonline-p.com/lib/0.1.1/js/msal.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
</head>
<body>
<script class="pre">
var userAgentApplication = new Msal.UserAgentApplication("b3dd78af-d9da-459d-bf01-f87104d87450", null, function (errorDes, token, error, tokenType) {
// this callback is called after loginRedirect OR acquireTokenRedirect (not used for loginPopup/aquireTokenPopup)
});
userAgentApplication.loginPopup(["user.read"]).then(function (token) {
var user = userAgentApplication.getUser();
console.log(user);
console.log('access token:' + token);
InvokeApi(token);
}, function (error) {
alert('login error:' + error);
});
function InvokeApi(token) {
$.ajax({
type: "GET",
url: "http://localhost:9184/api/values",
headers: {
'Authorization': 'Bearer ' + token,
},
}).done(function (data) {
console.log('invoked Web API \'http://localhost:9184/api/values\' with result: ' + data);
alert(data);
}).fail(function (jqXHR, textStatus) {
alert('Error getting data');
});
}
</script>
</body>
</html>
Result:
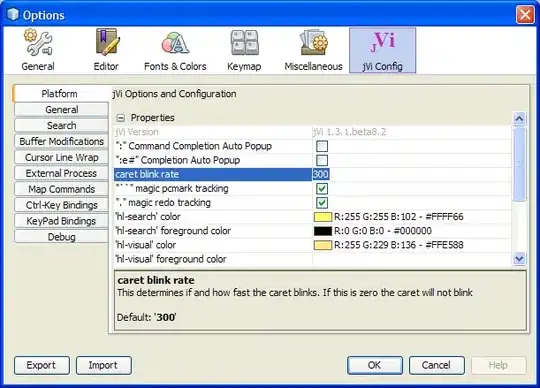
Additionally, you could refer to this git sample JavaScript Single Page Application with an ASP.NET backend, using msal.js.