With Python, Flask and MSAL 2.0 I did the following:
@app.route("/logout")
def logout():
logout_user()
if session.get("user"): # Used MS Login
# Wipe out user and its token cache from session
session.clear()
# Also logout from your tenant's web session
redirect(
Config.AUTHORITY
+ "/oauth2/v2.0/logout"
+ "?post_logout_redirect_uri="
+ url_for("login", _external=True, _scheme="https")
)
app.logger.info("Logging user out.")
return redirect(url_for("login"))
In Azure Portal configured my endpoints like this:
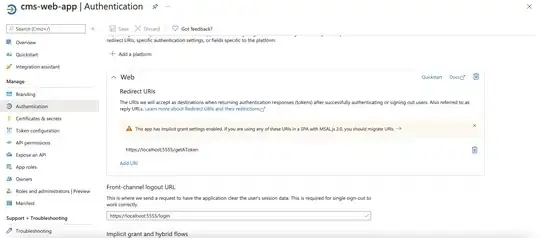
Environment variables are configured like this
# Oauth - Azure Active Directory and MSAL
export CLIENT_SECRET=<your-client-secret>
export CLIENT_ID=<your-client-id>
export REDIRECT_PATH=/getAToken
export SESSION_TYPE=filesystem
export AUTHORITY=https://login.microsoftonline.com/common
export SCOPE=User.Read
export SESSION_TYPE=filesystem
config.py file
import os
from dotenv import load_dotenv
basedir = os.path.abspath(os.path.dirname(__file__))
load_dotenv(os.path.join(basedir, ".env"))
class Config(object):
### Info for MS Authentication ###
### As adapted from: https://github.com/Azure-Samples/ms-identity-python-webapp ###
CLIENT_SECRET = os.environ.get("CLIENT_SECRET")
# In your production app, Microsoft recommends you to use other ways to store your secret,
# such as KeyVault, or environment variable as described in Flask's documentation here:
# https://flask.palletsprojects.com/en/1.1.x/config/#configuring-from-environment-variables
# CLIENT_SECRET = os.getenv("CLIENT_SECRET")
# if not CLIENT_SECRET:
# raise ValueError("Need to define CLIENT_SECRET environment variable")
AUTHORITY = os.environ.get("AUTHORITY") # For multi-tenant app, else put tenant name
# AUTHORITY = "https://login.microsoftonline.com/Enter_the_Tenant_Name_Here"
CLIENT_ID = os.environ.get("CLIENT_ID") #
REDIRECT_PATH = os.environ.get(
"REDIRECT_PATH"
) # Used to form an absolute URL; must match to app's redirect_uri set in AAD
# You can find the proper permission names from this document
# https://learn.microsoft.com/en-us/graph/permissions-reference
SCOPE = [os.environ.get("SCOPE")] # Only need to read user profile for this app
SESSION_TYPE = os.environ.get(
"SESSION_TYPE"
) # Token cache will be stored in server-side session