As per the documentation Document Object Model (DOM) Level 2 HTML Specification click()
method doesn't accepts any parameters, doesn't return any value and doesn't report any exceptions.
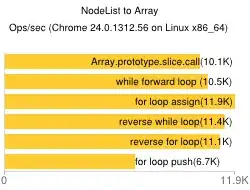
So, its clear that we can't override click()
method in the first place.
But you can always write a customized user function to click on a WebElement along with certain types of waits. In these cases Selenium's in-built Explicit Wait i.e. the WebDriverWait comes to our help.
ExplicitWait
As per the documentation, an ExplicitWait is a code block you define, configure and implement for the WebDriver instance to wait for a certain condition to be met before proceeding for the next line of code. There are some methods that helps us to implement ExplicitWait that will wait only as long as required. WebDriverWait in combination with ExpectedCondition is one of the way ExplicitWait can be achieved.
You can find a detailed discussion on ExplicitWait in this discussion.