If you mean you want the range of values to be scaled for each cvar
, you can do that before passing the data frame to ggplot:
library(dplyr)
daten2 <- daten %>%
group_by(cvar) %>%
# option 1: this scales the values at each varX from 0 to max
mutate(value.scaled = value / max(value)) %>%
# option 2: this scales the values at each varX from min to max
mutate(value.scaled2 = (value - min(value)) / (max(value) - min(value))) %>%
ungroup() %>%
mutate(setting = factor(setting,
levels = c("max", "upper", "center", "lower", "min")))
p1 <- ggplot(data = daten2,
aes(x = factor(cvar), y = value.scaled, group = setting, color = setting)) +
geom_line(size = 1) +
expand_limits(y = 0) +
coord_polar() +
ggtitle("Scaled value range from 0 to max")
p2 <- ggplot(data = daten2,
aes(x = factor(cvar), y = value.scaled2, group = setting, color = setting)) +
geom_line(size = 1) +
coord_polar() +
ggtitle("Scaled value range from min to max")
gridExtra::grid.arrange(p1, p2, nrow = 1)
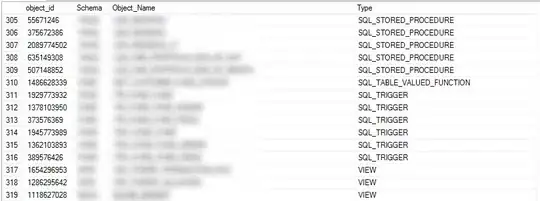
Edit: If you want to join the first and last cvar
values, you can repeat var1 at the end of the x-axis, and explicitly set expand = c(0, 0)
so that the last cvar
value joins up with the first under polar coordinates:
# I'm only demonstrating with one of the two cases above,
# but the same principle applies in either case.
ggplot(data = daten2 %>%
filter(cvar == "var1") %>% # make a copy of the dataset with only var1
mutate(cvar = "var6") %>%
rbind(daten2), # append the copy to the full dataset
aes(x = factor(cvar), y = value.scaled2, group = setting, color = setting)) +
geom_line(size = 1) +
scale_x_discrete(expand = c(0, 0),
breaks = c("var1", "var2", "var3", "var4", "var5")) +
coord_polar() +
ggtitle("Scaled value range from min to max")

And if you want the lines to be straight under polar coordinates, the answer here describes an alternative:
coord_radar <- function (theta = "x", start = 0, direction = 1) {
theta <- match.arg(theta, c("x", "y"))
r <- if (theta == "x") "y" else "x"
ggproto("CordRadar", CoordPolar, theta = theta, r = r, start = start,
direction = sign(direction),
is_linear = function(coord) TRUE)
}
ggplot(data = daten2 %>%
filter(cvar == "var1") %>%
mutate(cvar = "var6") %>%
rbind(daten2),
aes(x = factor(cvar), y = value.scaled2, group = setting, color = setting)) +
geom_line(size = 1) +
scale_x_discrete(expand = c(0, 0),
breaks = c("var1", "var2", "var3", "var4", "var5")) +
coord_radar() +
ggtitle("Scaled value range from min to max")
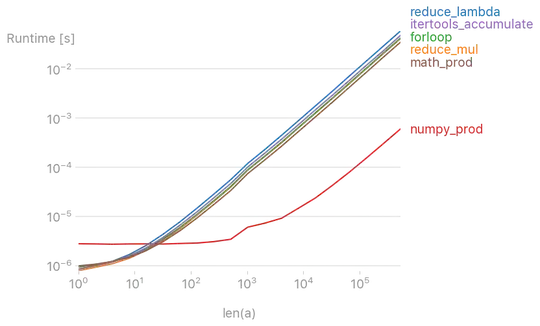