As this is a common symptom with many causes, it may help to examine it in isolation. Starting from the example seen here, the variation below listens to the combo's SelectionModel
and displays the value of the current selection in a TextField
. Note that the combo's selected index becomes -1 when the Clear button is used to clear the combo's model.
SingleSelectionModel selectionModel = comboBox.getSelectionModel();
TextField selection = new TextField(String.valueOf(selectionModel.getSelectedIndex()));
selectionModel.selectedIndexProperty().addListener((Observable o) -> {
selection.setText(String.valueOf(selectionModel.getSelectedIndex()));
});
…
dialogPane.setContent(new VBox(8, textField, datePicker, comboBox, selection, …));
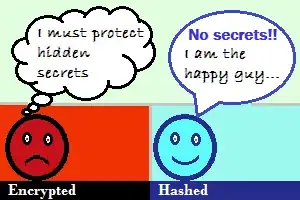
Complete example:
import java.time.LocalDate;
import java.util.Optional;
import javafx.application.Application;
import javafx.application.Platform;
import javafx.beans.Observable;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.ComboBox;
import javafx.scene.control.DatePicker;
import javafx.scene.control.Dialog;
import javafx.scene.control.DialogPane;
import javafx.scene.control.SingleSelectionModel;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
/**
* @see https://stackoverflow.com/a/46504588/230513
* @see http://stackoverflow.com/q/44147595/230513
* @see http://www.javaworld.com/article/2991463/
*/
public class DialogTest extends Application {
@Override
public void start(Stage primaryStage) {
Dialog<Results> dialog = new Dialog<>();
dialog.setTitle("Dialog Test");
dialog.setHeaderText("Please specify…");
DialogPane dialogPane = dialog.getDialogPane();
dialogPane.getButtonTypes().addAll(ButtonType.OK, ButtonType.CANCEL);
TextField textField = new TextField("Name");
DatePicker datePicker = new DatePicker(LocalDate.now());
ObservableList<Venue> options =
FXCollections.observableArrayList(Venue.values());
ComboBox<Venue> comboBox = new ComboBox<>(options);
SingleSelectionModel selectionModel = comboBox.getSelectionModel();
selectionModel.selectFirst();
TextField selection = new TextField(String.valueOf(selectionModel.getSelectedIndex()));
selectionModel.selectedIndexProperty().addListener((Observable o) -> {
selection.setText(String.valueOf(selectionModel.getSelectedIndex()));
});
Button clear = new Button("Clear");
clear.setOnAction((action) -> {
options.clear();
});
Button restore = new Button("Restore");
restore.setOnAction((action) -> {
options.clear();
options.addAll(Venue.values());
selectionModel.selectLast();
});
dialogPane.setContent(new VBox(8, textField, datePicker, comboBox, selection, clear, restore));
Platform.runLater(textField::requestFocus);
dialog.setResultConverter((ButtonType button) -> {
if (button == ButtonType.OK) {
return new Results(textField.getText(),
datePicker.getValue(), comboBox.getValue());
}
return null;
});
Optional<Results> optionalResult = dialog.showAndWait();
optionalResult.ifPresent((Results results) -> {
System.out.println(
results.text + " " + results.date + " " + results.venue);
});
}
private static enum Venue {Here, There, Elsewhere}
private static class Results {
String text;
LocalDate date;
Venue venue;
public Results(String name, LocalDate date, Venue venue) {
this.text = name;
this.date = date;
this.venue = venue;
}
}
public static void main(String[] args) {
launch(args);
}
}