Create 2 XML drawables
rect_colored.xml
<shape android:shape="rectangle"
xmlns:android="http://schemas.android.com/apk/res/android">
<solid android:color="@color/colorAccent"/>
<corners android:bottomRightRadius="5dp"
android:topRightRadius="5dp"/>
</shape>
rect_white.xml
<shape android:shape="rectangle"
xmlns:android="http://schemas.android.com/apk/res/android" >
<stroke android:color="@color/colorPrimaryDark"
android:width="2dp"/>
<corners android:radius="5dp"/>
</shape>
Position the drawables appropriately
This will work differently with different root views. I'm using ConstraintLayout
for simplicity
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:layout_width="0dp"
android:layout_height="40dp"
android:layout_marginRight="8dp"
app:layout_constraintRight_toRightOf="parent"
android:layout_marginLeft="8dp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toTopOf="parent"
android:layout_marginTop="8dp"
android:background="@drawable/rect_white"
android:id="@+id/frameLayout"
app:layout_constraintBottom_toBottomOf="parent"
android:layout_marginBottom="8dp">
<!--Content here-->
</FrameLayout>
<FrameLayout
android:layout_width="36dp"
android:layout_height="36dp"
android:layout_marginBottom="0dp"
android:layout_marginRight="2dp"
android:layout_marginTop="0dp"
android:background="@drawable/rect_colored"
app:layout_constraintBottom_toBottomOf="@+id/frameLayout"
app:layout_constraintRight_toRightOf="@+id/frameLayout"
app:layout_constraintTop_toTopOf="@+id/frameLayout">
<!--Content here-->
</FrameLayout>
</android.support.constraint.ConstraintLayout>
The output
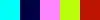