You can make the recursive approach perform a lot better by dividing through factors:
def choose(n, k):
if k == 0:
return 1
return n * choose(n-1, k-1) // k
This uses the recurrence relation:
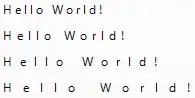
This approach will instantly compute 1000 C 800 without blowing the recursion stack. But, the underlying problem is still there - it's just postponed to much larger numbers. You may prefer to use one of the various closed-form solutions instead, for example:
from math import factorial
def choose(n, k):
if n < k:
n = k - n
return factorial(n) // (factorial(k) * factorial(n-k))