That is not your only problem.
Floating point numbers are not exact representations of all real numbers. In particular, they don't exactly represent numbers like square root two, and we can't even be sure that the floating point representation of a number like square root four will be exact.
There are at least two ways for a programmer to deal with this.
Solve that equation for y
. There are three solutions, each of which depends on this.
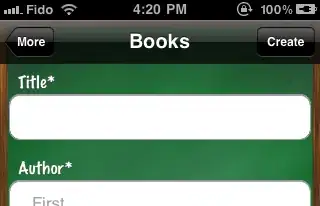
Just three roots, I would say. But lovely, exact ones. Use the sympy library if you want to do it in this way.
An inexact way that allows for floating point approximation, and that is applicable where you cannot solve the original equation is this one.
tolerance
is where the inexactitude comes in, being one's own estimate of how much difference one is willing to allow between an integer and a value that the square root routine produces. Notice that the abs
operator must be applied so that the difference is treated as a positive value.
tolerance = 0.0001
x = []
for y in range(-100, 101):
z = (16+y**2)**0.5
if abs(z-round(z)) < tolerance:
x.append(z)
print (x)
Result:
[5.0, 4.0, 5.0]