Generally speaking, if a function definition is in an expression position, then it's a function expression.
When is something an "expression position"? When you cannot put a statement there.
Arguments are expressions (you would not be able to pass an if
statement as an argument) therefore function name() {...}
is an expression in your example.
And because it is a function expression you could omit the name.
Now you might be wondering about the following: Assuming you know that 5 + 5
is an expression and that you can put 5 + 5
in a line just like so
5 + 5;
it seems we can put expression anywhere we want. So how does JavaScript decide, given
function name() {
//...
}
whether it is a function declaration or a function expression?
To answer this question we have to look at how the code is structured in general. At the top level, all you have is a list of statements:
<statement1>
<statement2>
<statement3>
...
There are various types of statements, an if
statement is one example. There is also the concept of an ExpressionStatement
. As the name implies, it's a statement that consists of a single expression. This is the rule that allows you to put 5 + 5;
in that list of statements.
But this parsing rule is restricted. It cannot start with the keyword function
(among others). Therefore, when JavaScript encounters the above function definition, it won't be parsed as a function expression, because it cannot parse it as an ExpressionStatement
. The only choice left is to parse it as a function declaration.
If you are curious about the structure of a JavaScript program you can use https://astexplorer.net (full disclosure: I built this site) to see how popular JavaScript parsers pare the program.
For example,
setTimeout(function name(){console.log(1)}, 1000)
is parsed to
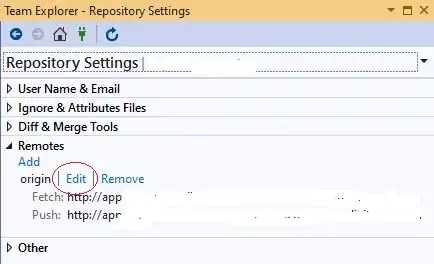
by acorn.
(you should really look at the live version though, because hovering over the AST highlights the corresponding source text, which makes things easier to understand)