There are a couple ways to do this:
1) HTML allows you to use the html <audio>
tag in most modern browsers for this very purpose
2) Use jQuery to create a new Audio
object (as you have already done) and pass in the url to the sound file as a parameter.
WARNING - example is quite loud by default
var audio = new Audio("https://www.computerhope.com/jargon/m/example.mp3");
$('#start').click(function() {
audio.play()
});
$('#pause').click(function() {
audio.pause()
});
.container
{
border: 1px solid black;
padding: 5px;
margin: 5px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<label>Using audio tag </label><br/>
<!-- use audio tag !-->
<audio id="playAudio" controls>
<source src="https://www.computerhope.com/jargon/m/example.mp3" />
</audio>
</div>
<div class="container">
<label>Using jQuery </label><br/>
<!-- add a button and handle this in jQuery !-->
<div>
<button id="start">play</button>
<button id="pause">pause</button>
</div>
</div>
Here is the full list of supported browsers for the <audio>
tag:
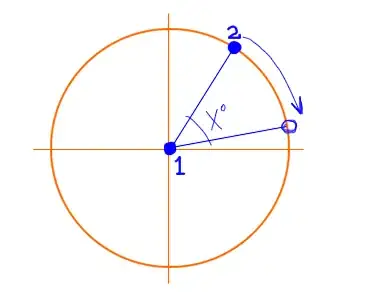
Audio credit to computerhope.com