This example demonstrates how arrow function names are assigned, and this behaviour cannot be changed. Arrow function name
is equal to the name of a variable or object property it was assigned to.
name
is non-writable property but is configurable in most implementations, including evergreen browsers, so it's safe to use for debugging purposes:
function namedArrow(name, fn) {
Object.defineProperty(fn, 'name', { enumerable: false, value: name });
return fn;
}
const foo = namedArrow('foo!', () => { throw new Error });
foo();
It outputs:
[![foo]](../../images/3794603084.webp)
For debugging purposes displayName
can be used also, which is supposed to play same role and is supported by some debuggers and browser debugger tools, notably Firefox and Chrome:
function namedArrow(name, fn) {
fn.displayName = name;
return fn;
}
It results in similar output, yet it doesn't play well with Error
call stack:
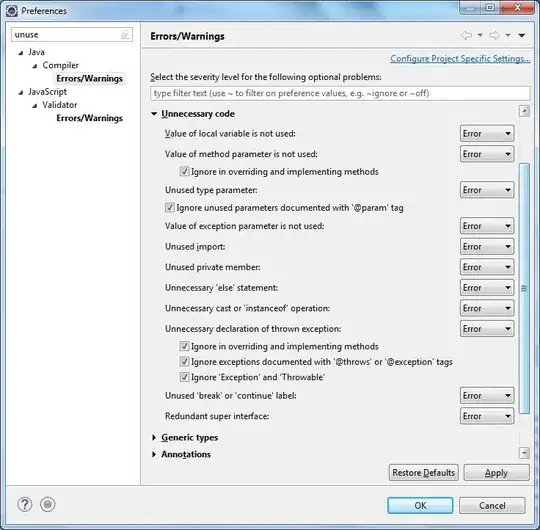