I tried my best to understand. First dividing the sepal length to the desired categories iris_limits
: "1-3","3-6","6-9"
iris$iris_limits <- cut(iris$Sepal.Length, c(1,3,6,9))
Note: no sepal length is in between 1-3, so you only have 2 groups.
Then you want each sepal length limit as a separate bar on the x axis, and each individual sepal length falling into category to be bar stacked onto each other? You linked to a stack bar chart with varying color for the stacked bars, is this what you want?
Create an ID for each sepal length:
iris$ID <- factor(1:nrow(iris))
Plot, set color=~ID
if you want different colors for the stacked bars:
library(plotly)
p <- plot_ly(iris, x = ~iris_limits, y = ~Sepal.Length, type = 'bar', color=~ID) %>%
layout(yaxis = list(title = 'Count'), barmode = 'stack')
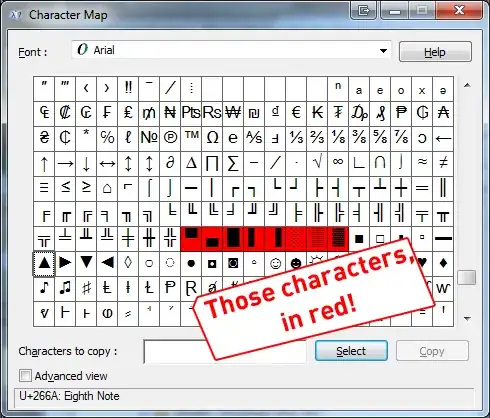
EDITED For version that is not stacked but grouped by iris_limits
, I switched to ggplot2
to make use of facet_wrap
functionality to segregate by iris_limits
, then use ggplotly
.
gg <- ggplot(iris, aes(x=ID, y=Sepal.Length, fill=iris_limits)) +
geom_bar(stat="identity", position="dodge") +
facet_wrap(~iris_limits, scales="free_x", labeller=label_both) +
theme_minimal() + xlab("") + ylab("Sepal Length") +
theme(axis.text.x=element_blank())
ggplotly(gg)
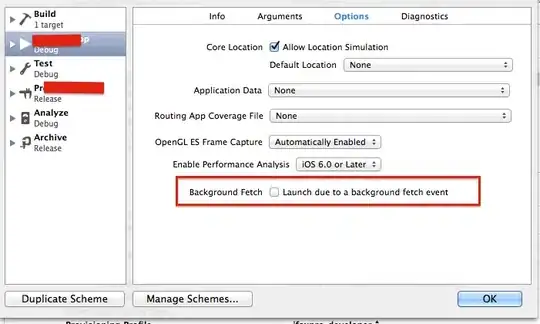
EDITED: Re: Changing legend title and tooltip display
To change the legend title, use labs
. Here it was also necessary to change the legend.title
font size under theme
to fit the ggplotly
margins.
To change the tooltip text, add text
parameter to aes
to create desired character string, then define aes
values to be displayed in tooltip
in ggplotly
.
gg <- ggplot(iris, aes(x=ID, y=Sepal.Length, fill=iris_limits,
text=paste("Sepal Length:", Sepal.Length, "cm"))) +
geom_bar(stat="identity", position="dodge") +
facet_wrap(~iris_limits, scales="free_x") +
theme_minimal() + xlab("") + ylab("Sepal Length (cm)") +
theme(axis.text.x=element_blank(), legend.title=element_text(size=10)) +
labs(fill="Sepal \nLength (cm)")
ggplotly(gg, tooltip=c("x", "text"))