You have two problems in the code you post.
1) You want to use samean
like a data frame--you want things like samean[, 1]
to work--but also like your new class shewhart
. So you don't want to replace the data.frame
class, you want to extend it. We change your line
# class(samean)<-"shewhart" # bad
class(samean) <- c("shewhart", class(samean)) # good
This way it is still a data.frame
, it's just a special type of data frame:
class(samean)
# [1] "shewhart" "data.frame"
2) For function arguments, use =
not <-
. This is a problem in your plot
command. Also, your function names its input x
, so you should refer to it only as x
inside the function.
# plot(x<-samean[,1],y<-samean[,2], type="b", col="blue",
xlim<-c(0,10), ylim<-c(120,140) ) # bad
plot(x = x[,1], y = x[,2], type = "b", col = "blue",
xlim = c(0,10), ylim = c(120,140) ) # good
With these changes, the code works as you describe. I re-formatted for readability, but the only real changes are the ones mentioned above.
aa <- c(1:10)
bb <- c(127, 128, 128.5, 125.5, 129, 126.5, 129, 126, 125, 130)
samean <- data.frame(aa, bb)
class(samean) <- c("shewhart", class(samean))
plot.shewhart <- function(x) {
mu = 127
sigma = 3.4
plot(
x = x[, 1],
y = x[, 2],
type = "b",
col = "blue",
xlim = c(0, 10),
ylim = c(120, 140)
)
abline(h = mu, col = "blue")
abline(h = mu + 3 * sigma / sqrt(100), col = "green")
abline(h = mu - 3 * sigma / sqrt(100), col = "green")
}
plot(samean)
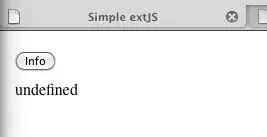
Please do not delete your question now that it has been answered.