In Java you can use Pattern Matching with a Regular Expression to gather the data you want from the URL String (or any string for that matter):
String urlString = "www.subdomain.domain.tld/page/?n=(sender_encoded_name)";
List<String> list = new ArrayList<>();
String regexString = "\\Q?n=(\\E(?s)(.*?)\\Q)\\E";
Pattern pattern = Pattern.compile("(?iu)" + regexString);
Matcher matcher = pattern.matcher(urlString);
while (matcher.find()) {
String match = matcher.group(1).trim();
list.add(match);
}
// Display all items found matching the supplied pattern
for(int i = 0; i < list.size(); i++) {
System.out.println(list.get(i));
}
Console output will be:
sender_encoded_name
To put this into a method you might have:
public List<String> getEncodedName(String urlString) {
List<String> list = new ArrayList<>();
String regexString = "\\Q?n=(\\E(?s)(.*?)\\Q)\\E";
Pattern pattern = Pattern.compile("(?iu)" + regexString);
Matcher matcher = pattern.matcher(urlString);
while (matcher.find()) {
String match = matcher.group(1).trim();
list.add(match);
}
return list;
}
And to use it:
String urlString = "www.subdomain.domain.tld/page/?n=(sender_encoded_name)";
List<String> list = getEncodedName(urlString);
// Display all items found matching the supplied pattern
for(int i = 0; i < list.size(); i++) {
System.out.println(list.get(i));
}
Console output will be:
sender_encoded_name
Regular Expression Explanation:
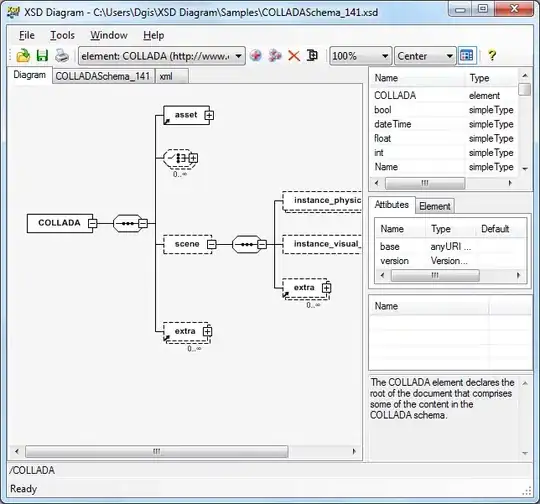