I have Created a Demo from your Code Reference.
First i have create a Model with 3 properties in it.
Model
public class DemoModel
{
public string Radio1 { get; set; }
public string Radio2 { get; set; }
public string Radio3 { get; set; }
}
After creating a Model i have created a Controller with name RadioRenderController in that controller i have added 4 Action Method in that Index returns Main View.
And Remaining 3 Action Methods [ GetView1 , GetView2 , GetView3 ] return partial View.
Controller
using System.Web.Mvc;
using WebApplication6.Models;
namespace WebApplication6.Controllers
{
public class RadioRenderController : Controller
{
// GET: RadioRender
public ActionResult Index()
{
DemoModel DemoModel = new Models.DemoModel();
return View(DemoModel);
}
public ActionResult GetView1()
{
return PartialView("_DemoView1");
}
public ActionResult GetView2()
{
return PartialView("_DemoView2");
}
public ActionResult GetView3()
{
return PartialView("_DemoView3");
}
}
}
View
@model WebApplication6.Models.DemoModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script type="text/javascript">
function GetView1() {
$("#viewPlaceHolder").load("/RadioRender/GetView1");
}
function GetView2() {
$("#viewPlaceHolder").load("/RadioRender/GetView2");
}
function GetView3() {
$("#viewPlaceHolder").load("/RadioRender/GetView3");
}
</script>
</head>
<body>
<div>
@Html.RadioButtonFor(m => m.Radio1, "1", new { @onclick = "GetView1();" }) Radio1
</div>
<div>
@Html.RadioButtonFor(m => m.Radio1, "2", new { @onclick = "GetView2();" }) Radio2
</div>
<div>
@Html.RadioButtonFor(m => m.Radio1, "3", new { @onclick = "GetView3();" }) Radio3
</div>
<div id="viewPlaceHolder"></div>
</body>
</html>
Partial Views

Output
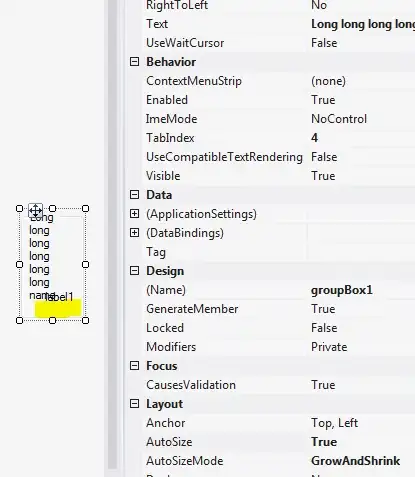