Create a subclass of NSWindow
and implement keyDown
event:
import Cocoa
import Carbon.HIToolbox
class CustomWindow: NSWindow {
override func keyDown(with event: NSEvent) {
switch Int(event.keyCode) {
case kVK_Escape:
print("Esc pressed")
default:
break
}
super.keyDown(with: event)
}
}
This line:
import Carbon.HIToolbox
Lets you use handy constants for keys, such as kVK_Escape
.
Set this class as your main window class in the Interface Builder and you're all set:
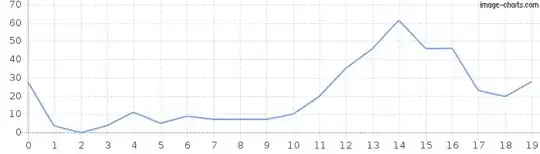
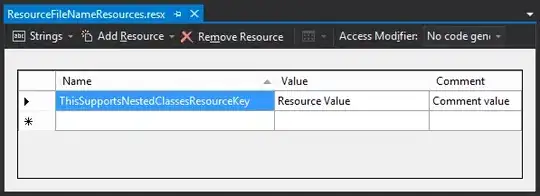
P.S. To do the same form NSViewController
, in viewDidLoad
do:
NSEvent.addLocalMonitorForEvents(matching: .keyDown) {
self.keyDown(with: $0)
return $0
}
P.P.S. To mute "bang" sound, don't call super upon Escape key press - move super call to default:
default:
super.keyDown(with: event)
EDIT:
If you don't want any sound on Escape key press, then the following approach should be used:
Make an NSView
subclass and set it to main view of the view controller:
import Cocoa
import Carbon.HIToolbox
class CustomView: NSView {
override func performKeyEquivalent(with event: NSEvent) -> Bool {
switch Int(event.keyCode) {
case kVK_Escape:
print("Esc pressed")
return true
default:
return super.performKeyEquivalent(with: event)
}
}
}