I believe sometimes you need to redeclare a variable to write less code.
One example is this function that generates a unique id:
function makeUniqueId(takenIds) {
do {
var id = Number.parseInt(Math.random() * 10);
} while (takenIds.includes(id))
}
Which may be invoked like that
makeUniqueId([1,2,3,4,5,6,7])
Here I declare id
variable simply inside do
block and it get's "hoisted" to the function scope. That would cause an error if I used let
, because while
block wouldn't see the variable from the do
block. Of course I could declate let
before do..while
, but that would create the same function scoped variable with extra line of code.
Another example is when you copypaste code to devtools console and every time variables get redeclared.
One more example. What if you want to keep your variable declarations close to their usages but still treat them as function globals? If you use let
in this fashion, you'll get rather confusing expirience in dev tools (all those Block, Block scopes).
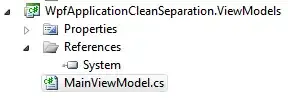
But var
'keeps' them together in one 'Local' list:
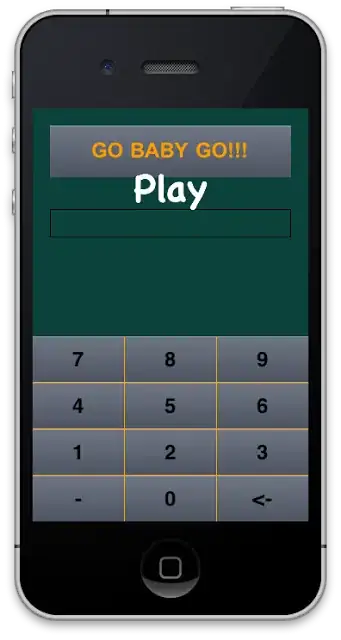