TL;NR: The following code works for gradle tasks, but the IDE does not understand that the app classes don't use Java 8.
In Android Studio 3.0.1 with gradle plugin version 3.0.1, the following works:
tasks.all {
task ->
if (task.name.endsWith('UnitTestJavaWithJavac')) {
android.compileOptions.setSourceCompatibility(JavaVersion.VERSION_1_8)
android.compileOptions.setTargetCompatibility(JavaVersion.VERSION_1_8)
}
}
}
Now I can write
@Test
public void throwNPE() {
assertThrows(NullPointerException.class, () ->
{ int [] nullarr = null; nullarr.clone(); }
);
}
and still keep my app code at Java 7 or even Java 6.
To check how Java compiler was actually configured, I can run javap
on my app classes and on my test classes:
javap -cp build/intermediates/classes/debug -verbose com.example.Main | grep major
javap -cp build/intermediates/classes/test/debug -verbose com.example.Tests | grep major
The results are:
major version: 51
major version: 52
Unfortunately, the IDE does not understand that the app classes don't use Java 8, and warns in the following situation (see this undocumented example of Java 8 incompatibility with Java 7):
interface I {}
public class Main implements I {
<T extends I> T getI() { return (T) this };
void foo(I value) {
System.out.println("foo(I)");
}
void foo(String value) {
System.out.println("foo(String)");
}
void warn() {
foo(getI());
}
}
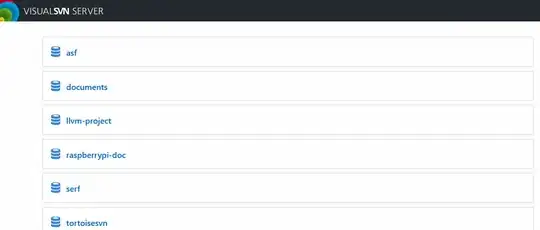
In spite of this warning, 'Make Proect' and 'Run Test' both pass.