Assuming you have checked the source code of the website already, If not here is one snippet which you are trying to get using JSOUP.
<div ng-controller="stockDetailSummary" ng-cloak>
<div class="stockDetail" ng-show="quoteFound">
<div ng-show="DataLoaded">
<h2>Summary</h2>
<div class="Column Left">
<div class="row">
<div class="label">
Open
</div>
<div class="value">
{{quoteObj.openPrc | number:2 }}
</div>
</div>
<div class="row alt">
<div class="label">
High
</div>
<div class="value">
{{quoteObj.high | number:2 }}
</div>
</div>
<div class="row">
<div class="label">
Low
</div>
<div class="value">
{{quoteObj.low | number:2 }}
</div>
If you see
<div class="value">
{{quoteObj.openPrc | number:2 }}
</div>
is returning what you are getting as output. Take it as String and do what so ever modification you want to do.
For your information the website is built on angularjs and the {{quoteObj.openPrc | number:2 }} are Angular pipes, a way to write display-value transformations that you can declare in your HTML.
EDIT:
As per your attached image, The data you want to retrieve is
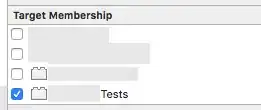
If you go into network tab of developer console you will find the exact request which is fetching the data
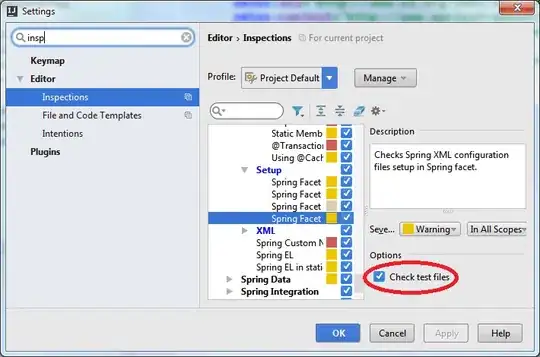
And corresponding response also you can see by navigating to preview tab

The same has been reffered in the html source code which you can see by pressing CTRL+U
<div class="stockDetail" ng-show="quoteFound">
<div ng-show="DataLoaded" class="">
<h2>Summary</h2>
<div class="Column Left">
<div class="row">
<div class="label">Open</div>
<div class="value ng-binding">0.00</div>
</div>
<div class="row alt">
<div class="label">High</div>
<div class="value ng-binding">0.00</div>
</div>
<div class="row">
<div class="label">Low</div>
<div class="value ng-binding">0.00</div>
</div>
<div class="row alt">
<div class="label">Volume</div>
<div class="value ng-binding">0</div>
</div>
<div class="row">
<div class="label">52-Week High</div>
<div class="value ng-binding">8.66</div>
</div>
<div class="row alt">
<div class="label">52-Week Low</div>
<div class="value ng-binding">1.90</div>
</div>
</div>
<div class="Column Right">
<div class="row">
<div class="label">Exchange</div>
<div class="value ng-binding">TOR</div>
</div>
<div class="row alt">
<div class="label">Currency</div>
<div class="value ng-binding">CAD</div>
</div>
<div class="row">
<div class="label">P/E</div>
<div class="value ng-binding">0.00</div>
</div>
<div class="row alt">
<div class="label">EPS</div>
<div class="value ng-binding">-0.01</div>
</div>
<div class="row">
<div class="label">Dividend</div>
<div class="value ng-binding">0.00</div>
</div>
<div class="row alt">
<div class="label">Yield</div>
<div class="value ng-binding">0.00<!-- ngIf: quoteObj.yield --></div>
</div>
</div>
<div class="clear"></div>
</div>
</div>
But here are few problems while fetching these values using JSOUP.
- JSOUP is a HTML Parser, It will not be able to fetch any content rendered by Javascript.
- The following Content is rendered by below api URL which is returning JSON data.
Request URL:https://data.bnn.ca/dispenser/bnnApi/quote/summary?s=ACB.TO
{
"statusCode": 200,
"generatedTimestamp": "2017-11-30T04:47:30.793-05:00",
"duration": 61,
"data": {
"stocks": [{
"symbol": "ACB.TO",
"currency": "CAD",
"trdprc": 0.0,
"volume": 0,
"dividend": 0.0,
"earnings": -0.01375,
"high": 0.0,
"low": 0.0,
"openPrc": 0.0,
"perRatio": 0.0,
"tradeDate": "29 NOV 2017",
"yield": 0.0,
"yearHigh": 8.66,
"yearLow": 1.9,
"exchange": "TOR"
}],
"invalidSymbols": []
}
}
So it will be better if you go through these links
Is there a way to embed a browser in Java?
https://stackoverflow.com/a/47485387/5313817