What i understand from the documentation :
The limit parameter controls the number of times the pattern is
applied and therefore affects the length of the resulting array. If
the limit n is greater than zero then the pattern will be applied at
most n - 1 times, the array's length will be no greater than n, and
the array's last entry will contain all input beyond the last matched
delimiter. If n is non-positive then the pattern will be applied as
many times as possible and the array can have any length. If n is zero
then the pattern will be applied as many times as possible, the array
can have any length, and trailing empty strings will be discarded.
This mean devise or cut it to n time on string s, so Lets analyse one by one to understand better :
Limit 1
String[] spl1 = str.split("o", 1);
This mean split it or cut it on just one string on the string o
in this case you will get all your input :
[boo:and:foo]
1
Limit 2
String[] spl1 = str.split("o", 2);
Which mean cut it one time on o
so i will put a break in the first o
boo:and:foo
-----^
in this case you will get two results :
[b,o:and:foo]
1 2
Limit 3
String[] spl1 = str.split("o", 3);
Which mean cut it two times on the first o
and on the second o
boo:and:foo
1----^^--------------2
in this case you will get three results :
[b, ,:and:foo]
1 2 3
Limit 4
String[] spl1 = str.split("o", 4);
Which mean cut it three times on the first, second and third o
boo:and:foo
1_____^^ ^
|___2 |___3
in this case you will get four results :
[b, ,:and:f,o]
1 2 3 4
Limit 5
String[] spl1 = str.split("o", 5);
Which mean cut it four times on first, second, third and forth o
boo:and:foo
1_____^^ ^^
|___2 ||___4
|____3
in this case you will get five results :
[b, ,:and:f, , ]
1 2 3 4 5
Just a simple animation to understand more :
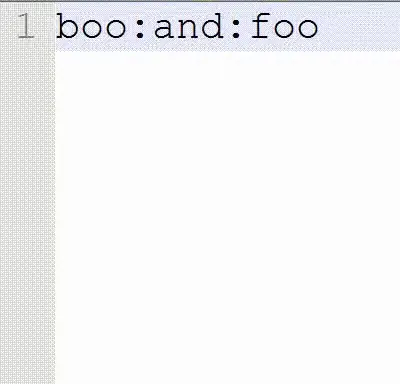