you json is not valid json structure for parsing , this not valid at all(check what is wrong with it here : https://jsonlint.com/)
not valid json string given by you
[
"pages":{
"FirstName": "Test1",
"LastName": "Test2",
"Address": "London, GB",
"Error": "Something's gone wrong"
},
{
"FirstName": "Test3",
"LastName": "Test4",
"Address": "NewYork, US",
"Error": "Something's gone wrong"
},
"labels": {
"DisplayName": "ContactNumber",
"Value": "01234 123 123"
}
}
]
However I fixed json you given
Fixed and working json
[{
"pages": [{
"FirstName": "Test1",
"LastName": "Test2",
"Address": "London, GB",
"Error": "Something's gone wrong"
},
{
"FirstName": "Test3",
"LastName": "Test4",
"Address": "NewYork, US",
"Error": "Something's gone wrong"
}
],
"labels": {
"DisplayName": "ContactNumber",
"Value": "01234 123 123"
}
}]
After applying fix json , your C# object is
public class Page
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string Address { get; set; }
public string Error { get; set; }
}
public class Labels
{
public string DisplayName { get; set; }
public string Value { get; set; }
}
public class RootObject
{
public List<Page> pages { get; set; }
public Labels labels { get; set; }
}
and I tried this with my fixed json and its working fine.
End of Edit
Below code is working fine for me with the class structure i provided you in below
for list
var jsonString = "[{ \"pages\": {\"id\": 12345, \"name\": \"John Doe\", \"number\": \"123\", \"test\": "+
"\"John\" }, \"labels\": { \"test\": \"test1\", \"test2\": \"test2\" } }," +
"{ \"pages\": {\"id\": 12345, \"name\": \"John Doe\", \"number\": \"123\", \"test\": "+
"\"John\" }, \"labels\": { \"test\": \"test1\", \"test2\": \"test2\" } }]";
var deserializedData = JsonConvert.DeserializeObject<List<RootObject>>(jsonString);
With single object as input
var jsonString = "{ \"pages\": {\"id\": 12345, \"name\": \"John Doe\", \"number\": \"123\", \"test\": "+
"\"John\" }, \"labels\": { \"test\": \"test1\", \"test2\": \"test2\" } }";
var deserializedData = JsonConvert.DeserializeObject<RootObject>(jsonString);
based on input
{ "pages": { "id": 12345, "name": "John Doe", "number": "123", "test":
"John" }, "labels": { "test": "test1", "test2": "test2" } }
below is generated classes
public class Pages
{
public int id { get; set; }
public string name { get; set; }
public string number { get; set; }
public string test { get; set; }
}
public class Labels
{
public string test { get; set; }
public string test2 { get; set; }
}
public class RootObject
{
public Pages pages { get; set; }
public Labels labels { get; set; }
}
If you have Json with you than you can generate C# class using visual studio itself.
In visual studio Find "Paste Sepcial" menu. i.e. copy you json string and click on Paste special , it will generate C# class for you.
you can follow my post : Generate Class From JSON or XML in Visual Studio
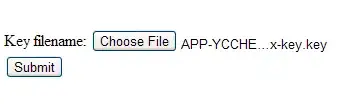
or use online website like : json2csharp