I achieved this effect by creating a SCNNode
with a SCNSphere
geometry and keeping it attached to the camera using ARSCNView.pointOfView
.
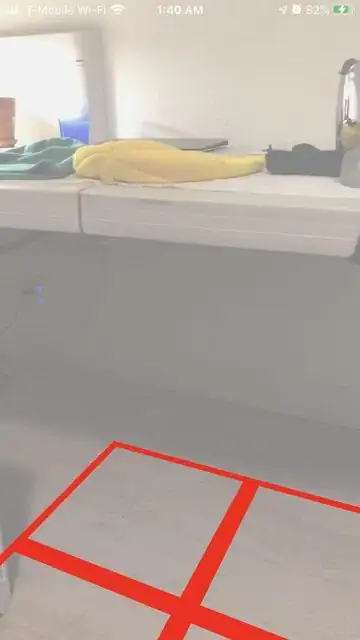
override func viewDidLoad() {
super.viewDidLoad()
let sphereFogNode = makeSphereNode()
arView.pointOfView!.addChildNode(sphereFogNode)
view.addSubview(arView)
}
private static func makeSphereGeom() -> SCNSphere {
let sphere = SCNSphere(radius: 5)
let material = SCNMaterial()
material.diffuse.contents = UIColor(white: 1.0, alpha: 0.7)
material.isDoubleSided = true
sphere.materials = [material]
return sphere
}
private static func makeSphereNode() -> SCNNode {
SCNNode(geometry: makeSphereGeom())
}
Clipping Outside Sphere
This darkens the camera along with anything outside the bounds of the sphere. Hit testing (ARFrame.hitTest
) does not respect the sphere boundary. You can receive results from outside the sphere.
Things which are outside your sphere will be seen through the sphere's opacity. It seems that non-transparent things will become transparent outside the sphere.
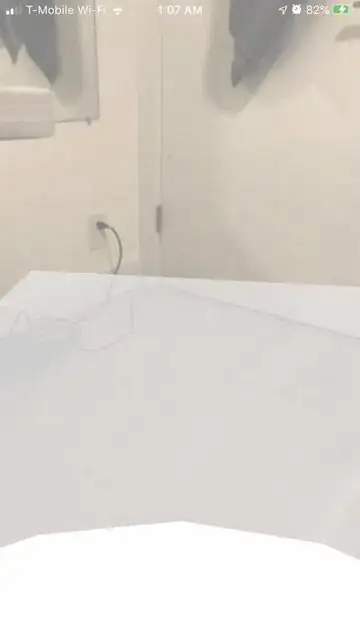
The white part is the plane inside a sphere and the grey is the plane outside the sphere. The plane is a solid white and non-transparent. I tried using SCNScene.fog*
to clip SceneKit graphics outside the sphere, but it seems that fog doesn't occlude rendered content, just affects its appearance. SCNCamera.zFar
doesn't work also as it clips based on the Z-distance, not on the straight line distance between the camera and target.
Just make your sphere big enough and everything will look fine.